private void UpdateDimensionAndGetCollectionPropertiesInfos(Dictionary <string, object> inputMaps, ref XmlDocument doc, ref XmlNodeList rows) { // note : dimension need to put on the top 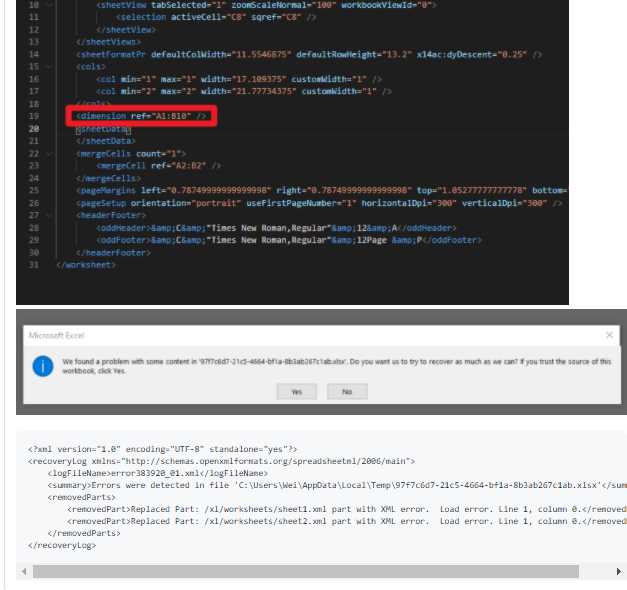 var dimension = doc.SelectSingleNode("/x:worksheet/x:dimension", _ns) as XmlElement; if (dimension == null) { throw new NotImplementedException("Excel Dimension Xml is null, please issue file for me. https://github.com/shps951023/MiniExcel/issues"); } var maxRowIndexDiff = 0; foreach (XmlElement row in rows) { // ==== get ienumerable infomation & maxrowindexdiff ==== //Type ienumerableGenricType = null; //IDictionary<string, PropertyInfo> props = null; //IEnumerable ienumerable = null; var xRowInfo = new XRowInfo(); xRowInfo.Row = row; XRowInfos.Add(xRowInfo); foreach (XmlElement c in row.SelectNodes($"x:c", _ns)) { var cr = c.GetAttribute("r"); var cLetter = new String(cr.Where(Char.IsLetter).ToArray()); c.SetAttribute("r", $"{cLetter}{{{{$rowindex}}}}"); var v = c.SelectSingleNode("x:v", _ns); if (v?.InnerText == null) { continue; } var matchs = (_isExpressionRegex.Matches(v.InnerText).Cast <Match>().GroupBy(x => x.Value).Select(varGroup => varGroup.First().Value)).ToArray(); var matchCnt = matchs.Length; var isMultiMatch = matchCnt > 1 || (matchCnt == 1 && v.InnerText != $"{{{{{matchs[0]}}}}}"); foreach (var formatText in matchs) { xRowInfo.FormatText = formatText; var propNames = formatText.Split('.'); if (propNames[0].StartsWith("$")) //e.g:"$rowindex" it doesn't need to check cell value type { continue; } if (!inputMaps.ContainsKey(propNames[0])) { throw new System.Collections.Generic.KeyNotFoundException($"Please check {propNames[0]} parameter, it's not exist."); } var cellValue = inputMaps[propNames[0]]; // 1. From left to right, only the first set is used as the basis for the list if (cellValue is IEnumerable && !(cellValue is string)) { xRowInfo.CellIEnumerableValues = cellValue as IEnumerable; // get ienumerable runtime type if (xRowInfo.IEnumerableGenricType == null) //avoid duplicate to add rowindexdiff  { var first = true; //TODO:if CellIEnumerableValues is ICollection or Array then get length or Count foreach (var element in xRowInfo.CellIEnumerableValues) //TODO: optimize performance? { if (xRowInfo.IEnumerableGenricType == null) { if (element != null) { xRowInfo.IEnumerablePropName = propNames[0]; xRowInfo.IEnumerableGenricType = element.GetType(); if (element is IDictionary <string, object> ) { xRowInfo.IsDictionary = true; var dic = element as IDictionary <string, object>; xRowInfo.PropsMap = dic.Keys.ToDictionary(key => key, key => dic[key] != null ? new PropInfo { UnderlyingTypePropType = Nullable.GetUnderlyingType(dic[key].GetType()) ?? dic[key].GetType() } : new PropInfo { UnderlyingTypePropType = typeof(object) }); } else { xRowInfo.PropsMap = xRowInfo.IEnumerableGenricType.GetProperties() .ToDictionary(s => s.Name, s => new PropInfo { PropertyInfo = s, UnderlyingTypePropType = Nullable.GetUnderlyingType(s.PropertyType) ?? s.PropertyType }); } } } // ==== get demension max rowindex ==== if (!first) //avoid duplicate add first one, this row not add status 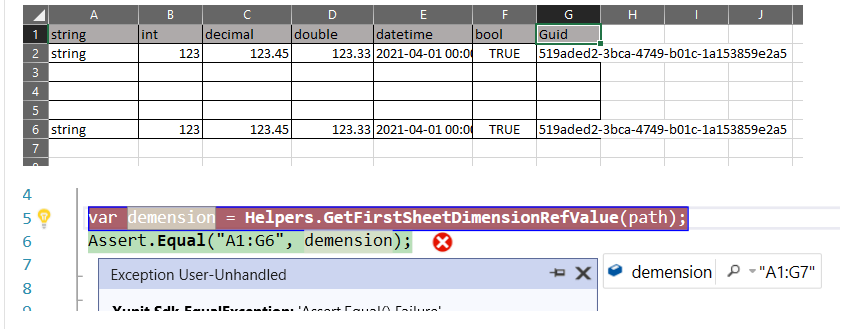 { maxRowIndexDiff++; } first = false; } } //TODO: check if not contain 1 index //only check first one match IEnumerable, so only render one collection at same row // auto check type https://github.com/shps951023/MiniExcel/issues/177 var prop = xRowInfo.PropsMap[propNames[1]]; var type = prop.UnderlyingTypePropType; //avoid nullable // if (!xRowInfo.PropsMap.ContainsKey(propNames[1])) { throw new InvalidDataException($"{propNames[0]} doesn't have {propNames[1]} property"); } if (isMultiMatch) { c.SetAttribute("t", "str"); } else if (Helpers.IsNumericType(type)) { c.SetAttribute("t", "n"); } else if (Type.GetTypeCode(type) == TypeCode.Boolean) { c.SetAttribute("t", "b"); } else if (Type.GetTypeCode(type) == TypeCode.DateTime) { c.SetAttribute("t", "str"); } break; } else if (cellValue is DataTable) { var dt = cellValue as DataTable; if (xRowInfo.CellIEnumerableValues == null) { xRowInfo.IEnumerablePropName = propNames[0]; xRowInfo.IEnumerableGenricType = typeof(DataRow); xRowInfo.IsDataTable = true; xRowInfo.CellIEnumerableValues = dt.Rows.Cast <DataRow>(); //TODO: need to optimize performance var first = true; foreach (var element in xRowInfo.CellIEnumerableValues) { // ==== get demension max rowindex ==== if (!first) //avoid duplicate add first one, this row not add status 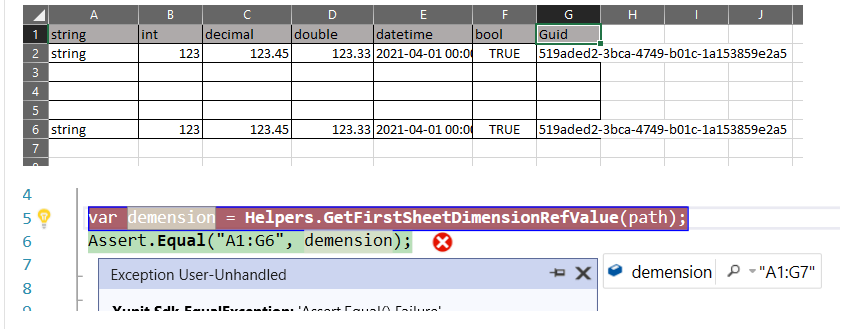 { maxRowIndexDiff++; } first = false; } //TODO:need to optimize //maxRowIndexDiff = dt.Rows.Count <= 1 ? 0 : dt.Rows.Count-1; xRowInfo.PropsMap = dt.Columns.Cast <DataColumn>().ToDictionary(col => col.ColumnName, col => new PropInfo { UnderlyingTypePropType = Nullable.GetUnderlyingType(col.DataType) } ); } var column = dt.Columns[propNames[1]]; var type = Nullable.GetUnderlyingType(column.DataType) ?? column.DataType; //avoid nullable if (!xRowInfo.PropsMap.ContainsKey(propNames[1])) { throw new InvalidDataException($"{propNames[0]} doesn't have {propNames[1]} property"); } if (isMultiMatch) { c.SetAttribute("t", "str"); } else if (Helpers.IsNumericType(type)) { c.SetAttribute("t", "n"); } else if (Type.GetTypeCode(type) == TypeCode.Boolean) { c.SetAttribute("t", "b"); } else if (Type.GetTypeCode(type) == TypeCode.DateTime) { c.SetAttribute("t", "str"); } } else { var cellValueStr = ExcelOpenXmlUtils.EncodeXML(cellValue); if (isMultiMatch) // if matchs count over 1 need to set type=str 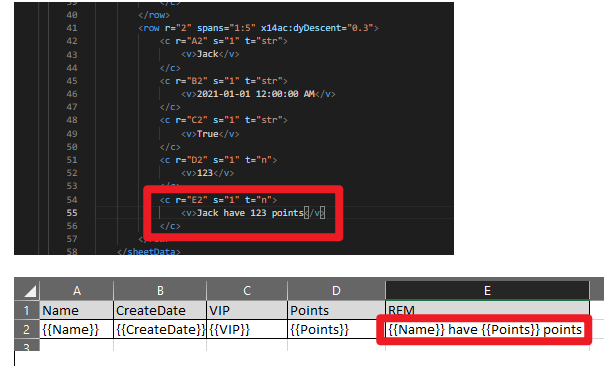 { c.SetAttribute("t", "str"); } else if (decimal.TryParse(cellValueStr, out var outV)) { c.SetAttribute("t", "n"); } else if (cellValue is bool) { c.SetAttribute("t", "b"); cellValueStr = (bool)cellValue ? "1" : "0"; } else if (cellValue is DateTime || cellValue is DateTime?) { //c.SetAttribute("t", "d"); cellValueStr = ((DateTime)cellValue).ToString("yyyy-MM-dd HH:mm:ss"); } v.InnerText = v.InnerText.Replace($"{{{{{propNames[0]}}}}}", cellValueStr); //TODO: auto check type and set value } } //if (xRowInfo.CellIEnumerableValues != null) //2. From left to right, only the first set is used as the basis for the list // break; } } // e.g <dimension ref=\"A1:B6\" /> only need to update B6 to BMaxRowIndex var @refs = dimension.GetAttribute("ref").Split(':'); var letter = new String(refs[1].Where(Char.IsLetter).ToArray()); var digit = int.Parse(new String(refs[1].Where(Char.IsDigit).ToArray())); dimension.SetAttribute("ref", $"{refs[0]}:{letter}{digit + maxRowIndexDiff}"); }
/// <summary> /// Check if we can correctly detect if the type has no value. /// </summary> private static bool IsTypeValid(Type type) { // TODO is this filtering neccessary? return(type != BoolType || Nullable.GetUnderlyingType(type) != null); }
public ModelDescription GetOrCreateModelDescription(Type modelType) { if (modelType == null) { throw new ArgumentNullException("modelType"); } Type underlyingType = Nullable.GetUnderlyingType(modelType); if (underlyingType != null) { modelType = underlyingType; } ModelDescription modelDescription; string modelName = ModelNameHelper.GetModelName(modelType); if (GeneratedModels.TryGetValue(modelName, out modelDescription)) { if (modelType != modelDescription.ModelType) { throw new InvalidOperationException( String.Format( CultureInfo.CurrentCulture, "A model description could not be created. Duplicate model name '{0}' was found for types '{1}' and '{2}'. " + "Use the [ModelName] attribute to change the model name for at least one of the types so that it has a unique name.", modelName, modelDescription.ModelType.FullName, modelType.FullName)); } return(modelDescription); } if (DefaultTypeDocumentation.ContainsKey(modelType)) { return(GenerateSimpleTypeModelDescription(modelType)); } if (modelType.IsEnum) { return(GenerateEnumTypeModelDescription(modelType)); } if (modelType.IsGenericType) { Type[] genericArguments = modelType.GetGenericArguments(); if (genericArguments.Length == 1) { Type enumerableType = typeof(IEnumerable <>).MakeGenericType(genericArguments); if (enumerableType.IsAssignableFrom(modelType)) { return(GenerateCollectionModelDescription(modelType, genericArguments[0])); } } if (genericArguments.Length == 2) { Type dictionaryType = typeof(IDictionary <,>).MakeGenericType(genericArguments); if (dictionaryType.IsAssignableFrom(modelType)) { return(GenerateDictionaryModelDescription(modelType, genericArguments[0], genericArguments[1])); } Type keyValuePairType = typeof(KeyValuePair <,>).MakeGenericType(genericArguments); if (keyValuePairType.IsAssignableFrom(modelType)) { return(GenerateKeyValuePairModelDescription(modelType, genericArguments[0], genericArguments[1])); } } } if (modelType.IsArray) { Type elementType = modelType.GetElementType(); return(GenerateCollectionModelDescription(modelType, elementType)); } if (modelType == typeof(NameValueCollection)) { return(GenerateDictionaryModelDescription(modelType, typeof(string), typeof(string))); } if (typeof(IDictionary).IsAssignableFrom(modelType)) { return(GenerateDictionaryModelDescription(modelType, typeof(object), typeof(object))); } if (typeof(IEnumerable).IsAssignableFrom(modelType)) { return(GenerateCollectionModelDescription(modelType, typeof(object))); } return(GenerateComplexTypeModelDescription(modelType)); }
public static bool IsNullable(this Type type) { return(Nullable.GetUnderlyingType(type) != null); }
/// <summary> /// 属性转换为列 /// </summary> /// <param name="column"></param> /// <param name="def"></param> /// <returns></returns> public string Property2Column(IColumnDescriptor column, out string def) { def = ""; var propertyType = column.PropertyInfo.PropertyType; var isNullable = propertyType.IsNullable(); if (isNullable) { propertyType = Nullable.GetUnderlyingType(propertyType); if (propertyType == null) throw new Exception("Property2Column error"); } if (propertyType.IsEnum) { if (!isNullable) { def = "DEFAULT 0"; } return "SMALLINT"; } if (propertyType.IsGuid()) return "UUID"; var typeCode = Type.GetTypeCode(propertyType); if (typeCode == TypeCode.String) { if (column.Max) return "TEXT"; if (column.Length < 1) return "VARCHAR(50)"; return $"VARCHAR({column.Length})"; } if (typeCode == TypeCode.Char) { return $"CHAR({column.Length})"; } if (typeCode == TypeCode.Boolean) { if (!isNullable) { def = "DEFAULT FALSE"; } return "boolean"; } if (typeCode == TypeCode.Byte) { if (!isNullable) { def = "DEFAULT 0"; } return "SMALLINT"; } if (typeCode == TypeCode.Int16) { if (column.IsPrimaryKey) { return "SMALLSERIAL"; } if (!isNullable) { def = "DEFAULT 0"; } return "SMALLINT"; } if (typeCode == TypeCode.Int32) { if (column.IsPrimaryKey) { return "SERIAL"; } if (!isNullable) { def = "DEFAULT 0"; } return "INTEGER"; } if (typeCode == TypeCode.Int64) { if (column.IsPrimaryKey) { return "BIGSERIAL"; } if (!isNullable) { def = "DEFAULT 0"; } return "BIGINT"; } if (typeCode == TypeCode.DateTime) { if (!isNullable) { def = "DEFAULT CURRENT_TIMESTAMP"; } return "TIMESTAMP"; } if (typeCode == TypeCode.Decimal || typeCode == TypeCode.Double || typeCode == TypeCode.Single) { if (!isNullable) { def = "DEFAULT 0"; } return "MONEY"; } return string.Empty; }
private static SqlDbType GetSqlDbType(Type type) { //Проверка, является ли переданный тип наследником BaseSmartCoreObject if (type.IsSubclassOf(typeof(BaseEntityObject))) { return(SqlDbType.Int); } if (type.IsEnum) { return(SqlDbType.SmallInt); } Type nullable = Nullable.GetUnderlyingType(type); if (nullable != null) { string typeName = nullable.Name.ToLower(); switch (typeName) { case "int32": return(SqlDbType.Int); case "int16": return(SqlDbType.SmallInt); case "datetime": return(SqlDbType.DateTime); case "bool": return(SqlDbType.Bit); case "boolean": return(SqlDbType.Bit); case "double": return(SqlDbType.Float); default: return(SqlDbType.NVarChar); } } else { string typeName = type.Name.ToLower(); switch (typeName) { case "averageutilization": return(SqlDbType.VarBinary); case "bool": return(SqlDbType.Bit); case "boolean": return(SqlDbType.Bit); case "byte[]": return(SqlDbType.VarBinary); case "datetime": return(SqlDbType.DateTime); case "componentdirectivethreshold": return(SqlDbType.VarBinary); case "directivethreshold": return(SqlDbType.VarBinary); case "trainingthreshold": return(SqlDbType.VarBinary); case "double": return(SqlDbType.Float); case "highlight": return(SqlDbType.Int); case "int16": return(SqlDbType.SmallInt); case "int32": return(SqlDbType.Int); case "lifelength": return(SqlDbType.VarBinary); case "maintenancedirectivethreshold": return(SqlDbType.VarBinary); case "string": return(SqlDbType.NVarChar); case "timespan": return(SqlDbType.Int); default: return(SqlDbType.NVarChar); } } }
private static ISchemaType CacheType(Type propType, ISchemaProvider schema, bool createEnumTypes, bool createNewComplexTypes) { if (propType.IsEnumerableOrArray()) { propType = propType.GetEnumerableOrArrayType(); } if (!schema.HasType(propType) && !ignoreTypes.Contains(propType.Name)) { var typeInfo = propType.GetTypeInfo(); string description = ""; var d = (DescriptionAttribute)typeInfo.GetCustomAttribute(typeof(DescriptionAttribute), false); if (d != null) { description = d.Description; } if (createNewComplexTypes && (typeInfo.IsClass || typeInfo.IsInterface)) { // add type before we recurse more that may also add the type // dynamcially call generic method // hate this, but want to build the types with the right Genenics so you can extend them later. // this is not the fastest, but only done on schema creation var method = schema.GetType().GetMethod("AddType", new[] { typeof(string), typeof(string) }); method = method.MakeGenericMethod(propType); var t = (ISchemaType)method.Invoke(schema, new object[] { propType.Name, description }); var fields = GetFieldsFromObject(propType, schema, createEnumTypes); t.AddFields(fields); return(t); } else if (createEnumTypes && typeInfo.IsEnum && !schema.HasType(propType.Name)) { var t = schema.AddEnum(propType.Name, propType, description); return(t); } else if (createEnumTypes && propType.IsNullableType() && Nullable.GetUnderlyingType(propType).GetTypeInfo().IsEnum&& !schema.HasType(Nullable.GetUnderlyingType(propType).Name)) { Type type = Nullable.GetUnderlyingType(propType); var t = schema.AddEnum(type.Name, type, description); return(t); } } else if (schema.HasType(propType.Name)) { return(schema.Type(propType.Name)); } return(null); }
public virtual DefaultExpressionOrValue ConvertSqlServerDefaultValue( [NotNull] Type propertyType, [NotNull] string sqlServerDefaultValue) { Check.NotNull(propertyType, nameof(propertyType)); Check.NotEmpty(sqlServerDefaultValue, nameof(sqlServerDefaultValue)); if (sqlServerDefaultValue.Length < 2) { return(null); } while (sqlServerDefaultValue[0] == '(' && sqlServerDefaultValue[sqlServerDefaultValue.Length - 1] == ')') { sqlServerDefaultValue = sqlServerDefaultValue.Substring(1, sqlServerDefaultValue.Length - 2); } if (string.IsNullOrEmpty(sqlServerDefaultValue)) { return(null); } if (_defaultValueIsExpression.IsMatch(sqlServerDefaultValue)) { return(new DefaultExpressionOrValue() { DefaultExpression = sqlServerDefaultValue }); } propertyType = propertyType.IsNullableType() ? Nullable.GetUnderlyingType(propertyType) : propertyType; if (typeof(string) == propertyType) { return(new DefaultExpressionOrValue() { DefaultValue = ConvertSqlServerStringLiteral(sqlServerDefaultValue) }); } if (typeof(bool) == propertyType) { return(new DefaultExpressionOrValue() { DefaultValue = ConvertSqlServerBitLiteral(sqlServerDefaultValue) }); } //TODO: decide what to do about byte[] default values try { return(new DefaultExpressionOrValue() { DefaultValue = Convert.ChangeType(sqlServerDefaultValue, propertyType) }); } catch (Exception) { return(null); } }
private static bool IsAnonymousTypeInternal(Type type) => type.IsConstructedGenericType && Nullable.GetUnderlyingType(type) == null && type.Name.Contains("<>") && type.Name.Contains("AnonymousType");
public CliParseResult <TParameters> Parse(string[] args) { var result = new TParameters(); bool showHelp = false; bool showVersion = false; var visitedArgs = new HashSet <OptionProperty>(); int argInd = 0; while (argInd < args.Length) { string arg = args[argInd]; if (!arg.StartsWith("-")) { _logger.Error($"Incorrect argument `{arg}`"); argInd++; continue; } string trimmedArg = arg.TrimStart('-'); if (trimmedArg == "help") { showHelp = true; argInd++; continue; } if (trimmedArg == "version") { showVersion = true; argInd++; continue; } OptionProperty?foundOption = OptionsProperties.FirstOrDefault(optionType => optionType.Option.ShortName == trimmedArg || optionType.LongName == trimmedArg); if (foundOption != null) { argInd++; string outValue = argInd < args.Length ? args[argInd] : ""; Type?underlyingType = Nullable.GetUnderlyingType(foundOption.PropertyInfo.PropertyType); var notNullableType = underlyingType != null ? underlyingType : foundOption.PropertyInfo.PropertyType; if (notNullableType == typeof(bool) && (argInd == args.Length || outValue.StartsWith("-") == true)) { CheckAndSetIfParsed(visitedArgs, result, foundOption, arg, true.ToString().ToLowerInvariant(), notNullableType); } else { CheckAndSetIfParsed(visitedArgs, result, foundOption, arg, outValue, notNullableType); argInd++; } } else { _logger.Error($"Unknown argument `{args[argInd]}`"); argInd++; } } foreach (OptionProperty optionProperty in OptionsProperties) { if (optionProperty.Option.Required) { if (!visitedArgs.Contains(optionProperty)) { _logger.Error($"Argument `{optionProperty.LongName}` should be set up"); } } } return(new CliParseResult <TParameters>(result, showHelp, showVersion)); }
public static object Value(Type t) { var x = Nullable.GetUnderlyingType(t); if (!(x is null)) { t = x; } if (t.IsArray) { return(Array(t.GetElementType())); } if (t.IsEnum) { return(Enum(t)); } if (t == typeof(string)) { return(String()); } if (t == typeof(char)) { return(Char()); } if (t == typeof(Color)) { return(Color()); } if (t == typeof(bool)) { return(Bool()); } if (t == typeof(DateTime)) { return(DateTime()); } if (t == typeof(decimal)) { return(Decimal()); } if (t == typeof(double)) { return(Double()); } if (t == typeof(float)) { return(Float()); } if (t == typeof(byte)) { return(UInt8()); } if (t == typeof(ushort)) { return(UInt16()); } if (t == typeof(uint)) { return(UInt32()); } if (t == typeof(ulong)) { return(UInt64()); } if (t == typeof(sbyte)) { return(Int8()); } if (t == typeof(short)) { return(Int16()); } if (t == typeof(int)) { return(Int32()); } if (t == typeof(long)) { return(Int64()); } if (t == typeof(TimeSpan)) { return(TimeSpan()); } if (t == typeof(char?)) { return(Char()); } if (t == typeof(Color?)) { return(Color()); } if (t == typeof(bool?)) { return(Bool()); } if (t == typeof(DateTime?)) { return(DateTime()); } if (t == typeof(decimal?)) { return(Decimal()); } if (t == typeof(double?)) { return(Double()); } if (t == typeof(float?)) { return(Float()); } if (t == typeof(byte?)) { return(UInt8()); } if (t == typeof(ushort?)) { return(UInt16()); } if (t == typeof(uint?)) { return(UInt32()); } if (t == typeof(ulong?)) { return(UInt64()); } if (t == typeof(sbyte?)) { return(Int8()); } if (t == typeof(short?)) { return(Int16()); } if (t == typeof(int?)) { return(Int32()); } if (t == typeof(long?)) { return(Int64()); } if (t == typeof(TimeSpan)) { return(TimeSpan()); } return(Object(t)); }
public static Type UnwrapNullableType(this Type type) => Nullable.GetUnderlyingType(type) ?? type;
public object ConvertObject(object obj, Type type) { if (type == null) { return(obj); } if (obj == null) { return(type.IsValueType ? Activator.CreateInstance(type) : null); } Type underlyingType = Nullable.GetUnderlyingType(type); if (type.IsInstanceOfType(obj)) // 如果待转换对象的类型与目标类型兼容,则无需转换 { return(obj); } else if ((underlyingType ?? type).IsEnum) // 如果待转换的对象的基类型为枚举 { if (underlyingType != null && string.IsNullOrEmpty(obj.ToString())) // 如果目标类型为可空枚举,并且待转换对象为null 则直接返回null值 { return(null); } else { return(Enum.Parse(underlyingType ?? type, obj.ToString())); } } else if (typeof(IConvertible).IsAssignableFrom(underlyingType ?? type)) // 如果目标类型的基类型实现了IConvertible,则直接转换 { try { return(Convert.ChangeType(obj, underlyingType ?? type, null)); } catch { return(underlyingType == null?Activator.CreateInstance(type) : null); } } else { TypeConverter converter = TypeDescriptor.GetConverter(type); if (converter.CanConvertFrom(obj.GetType())) { return(converter.ConvertFrom(obj)); } ConstructorInfo constructor = type.GetConstructor(Type.EmptyTypes); if (constructor != null) { object o = constructor.Invoke(null); PropertyInfo[] propertys = type.GetProperties(); Type oldType = obj.GetType(); foreach (PropertyInfo property in propertys) { PropertyInfo p = oldType.GetProperty(property.Name); if (property.CanWrite && p != null && p.CanRead) { property.SetValue(o, ConvertObject(p.GetValue(obj, null), property.PropertyType), null); } } return(o); } } return(obj); }
private void RenderRowsAndCells(Stream stream, XmlDocument doc, XmlNode sheetData) { //Q.Why so complex? //A.Because try to use string stream avoid OOM when rendering rows sheetData.RemoveAll(); sheetData.InnerText = "{{{{{{split}}}}}}"; //TODO: bad smell var prefix = string.IsNullOrEmpty(sheetData.Prefix) ? "" : $"{sheetData.Prefix}:"; var endPrefix = string.IsNullOrEmpty(sheetData.Prefix) ? "" : $":{sheetData.Prefix}"; //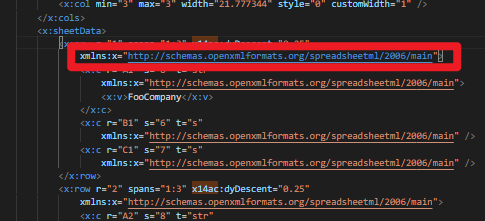 var contents = doc.InnerXml.Split(new string[] { $"<{prefix}sheetData>{{{{{{{{{{{{split}}}}}}}}}}}}</{prefix}sheetData>" }, StringSplitOptions.None);; using (var writer = new StreamWriter(stream, Encoding.UTF8)) { writer.Write(contents[0]); writer.Write($"<{prefix}sheetData>"); // prefix problem int originRowIndex; int rowIndexDiff = 0; foreach (var xInfo in XRowInfos) { var row = xInfo.Row; //TODO: some xlsx without r originRowIndex = int.Parse(row.GetAttribute("r")); var newRowIndex = originRowIndex + rowIndexDiff; if (xInfo.CellIEnumerableValues != null) { var first = true; foreach (var item in xInfo.CellIEnumerableValues) { var newRow = row.Clone() as XmlElement; newRow.SetAttribute("r", newRowIndex.ToString()); newRow.InnerXml = row.InnerXml.Replace($"{{{{$rowindex}}}}", newRowIndex.ToString()); if (xInfo.IsDictionary) { var dic = item as IDictionary <string, object>; foreach (var propInfo in xInfo.PropsMap) { var key = $"{{{{{xInfo.IEnumerablePropName}.{propInfo.Key}}}}}"; if (item == null) //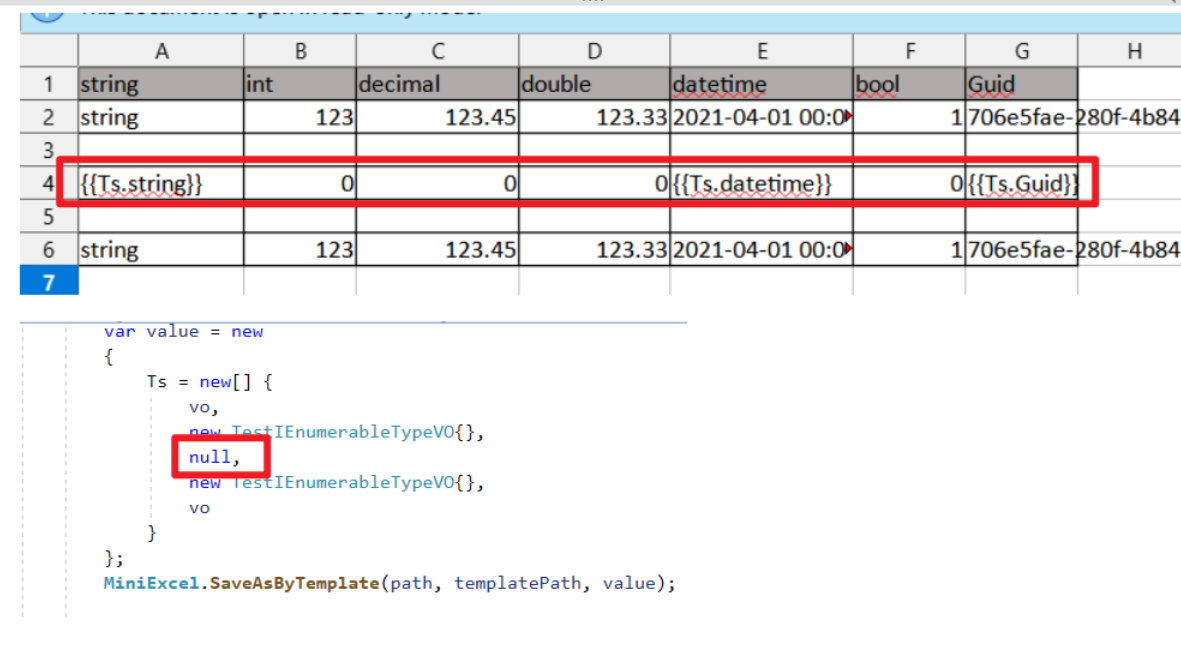 { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValue = dic[propInfo.Key]; if (cellValue == null) { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValueStr = ExcelOpenXmlUtils.EncodeXML(cellValue); var type = propInfo.Value.UnderlyingTypePropType; if (type == typeof(bool)) { cellValueStr = (bool)cellValue ? "1" : "0"; } else if (type == typeof(DateTime)) { //c.SetAttribute("t", "d"); cellValueStr = ((DateTime)cellValue).ToString("yyyy-MM-dd HH:mm:ss"); } //TODO: 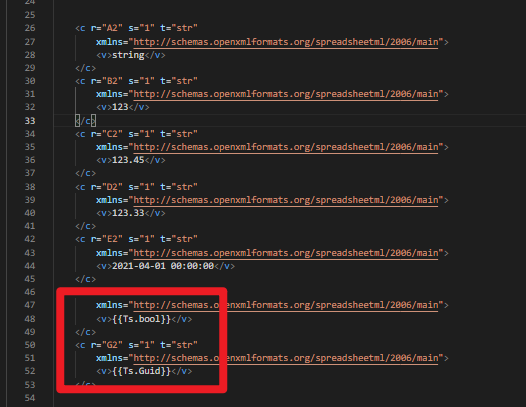 newRow.InnerXml = newRow.InnerXml.Replace(key, cellValueStr); } } else if (xInfo.IsDataTable) { var datarow = item as DataRow; foreach (var propInfo in xInfo.PropsMap) { var key = $"{{{{{xInfo.IEnumerablePropName}.{propInfo.Key}}}}}"; if (item == null) //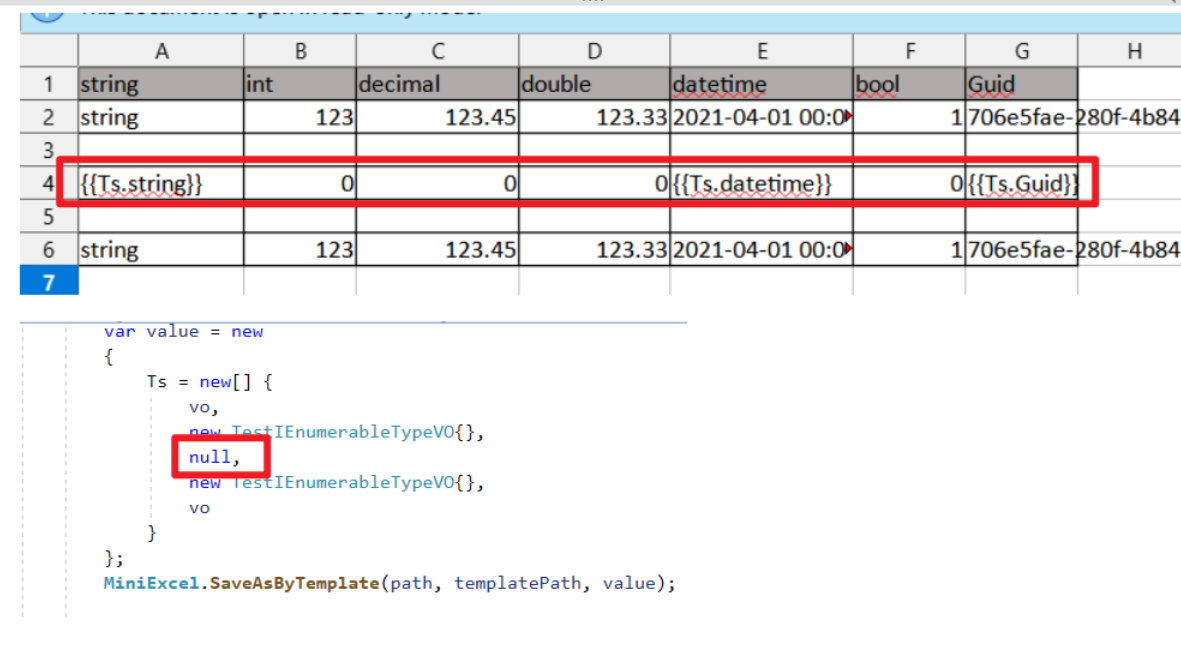 { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValue = datarow[propInfo.Key]; if (cellValue == null) { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValueStr = ExcelOpenXmlUtils.EncodeXML(cellValue); var type = propInfo.Value.UnderlyingTypePropType; if (type == typeof(bool)) { cellValueStr = (bool)cellValue ? "1" : "0"; } else if (type == typeof(DateTime)) { //c.SetAttribute("t", "d"); cellValueStr = ((DateTime)cellValue).ToString("yyyy-MM-dd HH:mm:ss"); } //TODO: 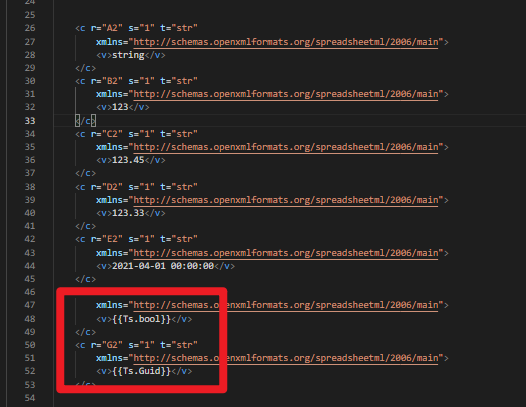 newRow.InnerXml = newRow.InnerXml.Replace(key, cellValueStr); } } else { foreach (var propInfo in xInfo.PropsMap) { var prop = propInfo.Value.PropertyInfo; var key = $"{{{{{xInfo.IEnumerablePropName}.{prop.Name}}}}}"; if (item == null) //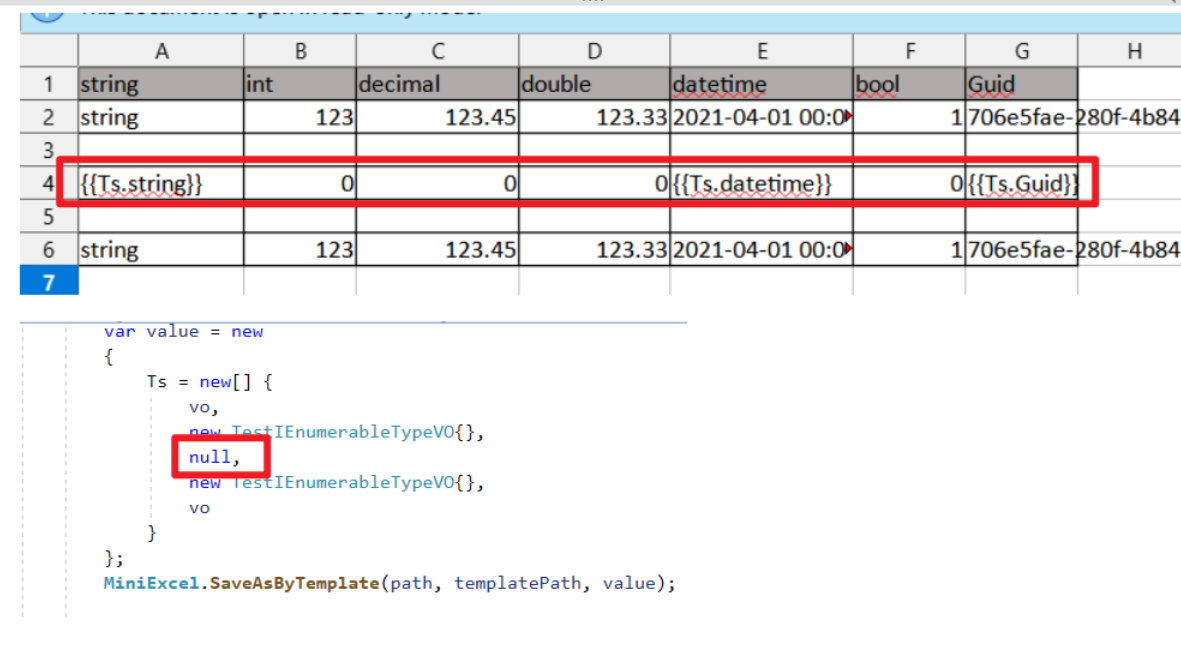 { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValue = prop.GetValue(item); if (cellValue == null) { newRow.InnerXml = newRow.InnerXml.Replace(key, ""); continue; } var cellValueStr = ExcelOpenXmlUtils.EncodeXML(cellValue); var type = Nullable.GetUnderlyingType(prop.PropertyType) ?? prop.PropertyType; if (type == typeof(bool)) { cellValueStr = (bool)cellValue ? "1" : "0"; } else if (type == typeof(DateTime)) { //c.SetAttribute("t", "d"); cellValueStr = ((DateTime)cellValue).ToString("yyyy-MM-dd HH:mm:ss"); } //TODO: 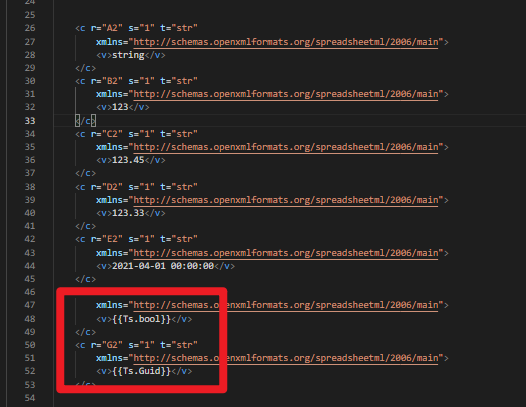 newRow.InnerXml = newRow.InnerXml.Replace(key, cellValueStr); } } // note: only first time need add diff 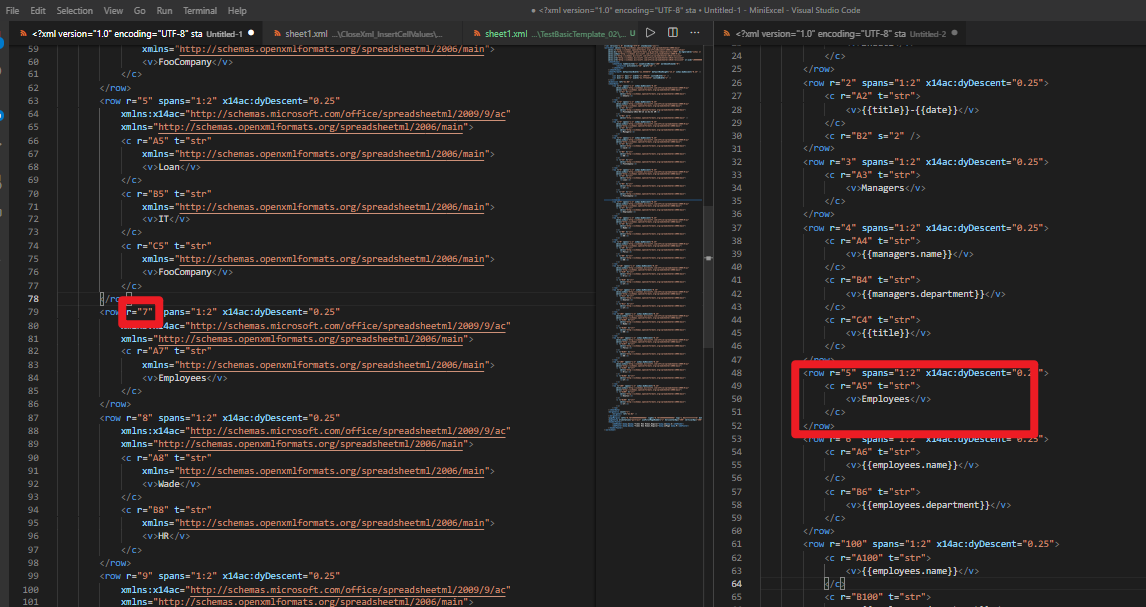 if (!first) { rowIndexDiff++; } first = false; newRowIndex++; writer.Write(CleanXml(newRow.OuterXml, endPrefix)); newRow = null; } } else { row.SetAttribute("r", newRowIndex.ToString()); row.InnerXml = row.InnerXml.Replace($"{{{{$rowindex}}}}", newRowIndex.ToString()); writer.Write(CleanXml(row.OuterXml, endPrefix)); } } writer.Write($"</{prefix}sheetData>"); writer.Write(contents[1]); } }
static CodeMemberMethod CreateMethod(string methodName, string defineName, ParameterInfo[] arguments, Type returnType) { var callMethod = new CodeMemberMethod(); callMethod.Attributes = MemberAttributes.Public | MemberAttributes.Final; callMethod.Name = methodName; callMethod.Parameters.AddRange(arguments.Select(a => new CodeParameterDeclarationExpression(a.ParameterType, a.Name)).ToArray()); callMethod.ReturnType = new CodeTypeReference(returnType); var tcpClientRef = new CodeFieldReferenceExpression(new CodeThisReferenceExpression(), NameOfClientField); var symbolNameRef = new CodeObjectCreateExpression(typeof(Symbol), new CodePrimitiveExpression(defineName)); var listBuilderParams = new List<CodeExpression>(); listBuilderParams.Add(symbolNameRef); foreach (var arg in arguments) { //************************************************** //** TODO AT THIS LINE SHOULD BE THE SERIALIZER ** //************************************************** if (BinFormater.IsPrimitive(arg.ParameterType) || IsNullablePrimitive(arg.ParameterType)) { listBuilderParams.Add(new CodeArgumentReferenceExpression(arg.Name)); } else { listBuilderParams.Add( new CodeMethodInvokeExpression( new CodeMethodReferenceExpression( new CodeTypeReferenceExpression(typeof(OSerializer)) , nameof(OSerializer.SerializeWithQuote)) , new CodeArgumentReferenceExpression(arg.Name))); } } var consLst = new CodeMethodInvokeExpression(new CodeTypeReferenceExpression(typeof(Cons)), nameof(Cons.List), listBuilderParams.ToArray()); var invoke = new CodeMethodInvokeExpression(new CodeMethodReferenceExpression(tcpClientRef, nameof(ISomeClient.Call)), consLst); // IS VOID METHOD if (returnType == typeof(void)) { callMethod.Statements.Add(invoke); } else { const string requestResultVarName = "requestResult"; callMethod.Statements.Add( new CodeVariableDeclarationStatement(typeof(object), requestResultVarName, invoke)); var requestResultRef = new CodeVariableReferenceExpression(requestResultVarName); var eql = new CodeBinaryOperatorExpression(requestResultRef, CodeBinaryOperatorType.ValueEquality, new CodePrimitiveExpression(null)); //**************************************************** //** TODO AT THIS LINE SHOULD BE THE DESERIALIZER ** //**************************************************** CodeExpression prepareResult = null; if (BinFormater.IsPrimitive(returnType) || IsNullablePrimitive(returnType)) { prepareResult = requestResultRef; } else { prepareResult = new CodeMethodInvokeExpression( new CodeTypeReferenceExpression(typeof(OSerializer)) , nameof(OSerializer.Deserialize) , new CodeCastExpression (typeof(Cons), requestResultRef) , new CodeTypeOfExpression(returnType)); } var castType = new CodeCastExpression(returnType, prepareResult); CodeStatement returnDefaultValue = null; if (returnType.IsValueType && Nullable.GetUnderlyingType(returnType) == null) { returnDefaultValue = new CodeMethodReturnStatement(new CodeObjectCreateExpression(returnType)); } else { returnDefaultValue = new CodeMethodReturnStatement(new CodePrimitiveExpression(null)); } var conditionalStatement = new CodeConditionStatement(eql, new[] {returnDefaultValue}, new[] {new CodeMethodReturnStatement(castType)}); callMethod.Statements.Add(conditionalStatement); } return callMethod; }
private static bool VerifyMapperResultHelper(this Mapper ultraMapper, object source, object target, ReferenceTracking referenceTracking) { //sharing the same reference or both null if (Object.ReferenceEquals(source, target)) { return(true); } //either source or target is null if (source == null || target == null) { return(false); } Type sourceType = source.GetType(); Type targetType = target.GetType(); if (!sourceType.IsValueType && source != null) { if (referenceTracking.Contains(source, targetType)) { return(true); } if (!sourceType.IsValueType) { referenceTracking.Add(source, targetType, target); } } //same value type: just compare their values if (sourceType == targetType && sourceType.IsBuiltInType(false)) { return(source.Equals(target)); } if (sourceType.IsEnumerable() && targetType.IsEnumerable()) { var firstPos = (source as IEnumerable).GetEnumerator(); var secondPos = (target as IEnumerable).GetEnumerator(); var hasFirst = firstPos.MoveNext(); var hasSecond = secondPos.MoveNext(); while (hasFirst && hasSecond) { if (!VerifyMapperResultHelper(ultraMapper, firstPos.Current, secondPos.Current, referenceTracking)) { return(false); } hasFirst = firstPos.MoveNext(); hasSecond = secondPos.MoveNext(); } if (hasFirst ^ hasSecond) { return(false); } } var typeMapping = ultraMapper.MappingConfiguration[ source.GetType(), target.GetType()]; foreach (var mapping in typeMapping.MemberMappings.Values) { if (mapping.MappingResolution == Internals.MappingResolution.RESOLVED_BY_CONVENTION && typeMapping.IgnoreMemberMappingResolvedByConvention) { continue; } var sourceValue = mapping.SourceMember .ValueGetter.Compile().DynamicInvoke(source); var converter = mapping.CustomConverter; if (converter != null) { sourceValue = converter.Compile().DynamicInvoke(sourceValue); } var targetValue = mapping.TargetMember .ValueGetter.Compile().DynamicInvoke(target); if (!mapping.SourceMember.MemberType.IsValueType && sourceValue != null) { if (referenceTracking.Contains(sourceValue, mapping.TargetMember.MemberType)) { continue; } var result = VerifyMapperResultHelper(ultraMapper, sourceValue, targetValue, referenceTracking); if (!result) { return(false); } referenceTracking.Add(sourceValue, mapping.TargetMember.MemberType, targetValue); } if (Object.ReferenceEquals(sourceValue, targetValue)) { continue; } bool isSourcePropertyNullable = Nullable.GetUnderlyingType(mapping.SourceMember.MemberType) != null; bool isTargetPropertyNullable = Nullable.GetUnderlyingType(mapping.TargetMember.MemberType) != null; if (isSourcePropertyNullable && !isTargetPropertyNullable && sourceValue == null && targetValue.Equals(mapping.TargetMember.MemberType.GetDefaultValueViaActivator())) { continue; } if (sourceValue == null ^ targetValue == null) { return(false); } if (sourceValue.GetType().IsEnumerable() && targetValue.GetType().IsEnumerable()) { var firstPos = (sourceValue as IEnumerable).GetEnumerator(); var secondPos = (targetValue as IEnumerable).GetEnumerator(); var hasFirst = firstPos.MoveNext(); var hasSecond = secondPos.MoveNext(); while (hasFirst && hasSecond) { if (!VerifyMapperResultHelper(ultraMapper, firstPos.Current, secondPos.Current, referenceTracking)) { return(false); } hasFirst = firstPos.MoveNext(); hasSecond = secondPos.MoveNext(); } if (hasFirst ^ hasSecond) { return(false); } } //same value type: just compare their values if (sourceValue.GetType() == targetValue.GetType() && sourceValue.GetType().IsValueType) { if (!sourceValue.Equals(targetValue)) { return(false); } } } return(true); }
static bool IsNullablePrimitive(Type t) { return IsNullable(t) && BinFormater.IsPrimitive(Nullable.GetUnderlyingType(t)); }
private static bool CanConvertFromString(Type destinationType) { destinationType = Nullable.GetUnderlyingType(destinationType) ?? destinationType; return(IsSimpleType(destinationType) || TypeDescriptor.GetConverter(destinationType).CanConvertFrom(typeof(string))); }
//#endregion #region public static void Fill(Type type, object value) public static object GetValue(Type type, object value) { //Проверка, является ли переданный тип наследником BaseSmartCoreObject if (type.IsSubclassOf(typeof(AbstractDictionary))) { try { var typeDict = _casEnvironment.GetDictionary(type); return(typeDict == null ? null : typeDict.GetItemById(ToInt32(value))); } catch (Exception ex) { throw ex; } } if (type.IsSubclassOf(typeof(StaticDictionary))) { try { //поиск в типе своиства Items PropertyInfo itemsProp = type.GetProperty("Items"); //поиск у типа конструктора беза параметров ConstructorInfo ci = type.GetConstructor(new Type[0]); //создание экземпляра статического словаря //(при этом будут созданы все его статические элементы, //которые будут доступны через статическое своиство Items) StaticDictionary instance = (StaticDictionary)ci.Invoke(null); //Получение элементов статического своиства Items IEnumerable staticList = (IEnumerable)itemsProp.GetValue(instance, null); int id = ToInt32(value); StaticDictionary res = staticList.Cast <StaticDictionary>().FirstOrDefault(o => o.ItemId == id) ?? staticList.Cast <StaticDictionary>().FirstOrDefault(o => o.ItemId == -1); return(res); } catch (Exception) { return(null); } } if (type.IsEnum) { try { object o = Enum.Parse(type, value.ToString()); return(o); } catch (Exception) { return(null); } } if (type.Name == typeof(Aircraft).Name) { try { var aircraft = _aircraftsCore.GetAircraftById(ToInt32(value)); return(aircraft); } catch (Exception) { return(null); } } if (type.Name == typeof(BaseComponent).Name) { try { BaseComponent bd = _casEnvironment.BaseComponents.GetItemById(ToInt32(value));//TODO(Evgenii Babak): использовать ComponentCore return(bd); } catch (Exception) { return(null); } } if (type.Name == typeof(Hangar).Name) { try { Hangar hangar = _casEnvironment.Hangars.GetItemById(ToInt32(value)); return(hangar); } catch (Exception) { return(null); } } if (type.Name == typeof(Operator).Name) { try { Operator op = _casEnvironment.Operators.GetItemById(ToInt32(value)); return(op); } catch (Exception) { return(null); } } if (type.Name == typeof(Store).Name) { try { Store store = _casEnvironment.Stores.GetItemById(ToInt32(value)); return(store); } catch (Exception) { return(null); } } if (type.Name == typeof(WorkShop).Name) { try { WorkShop workShop = _casEnvironment.WorkShops.GetItemById(ToInt32(value)); return(workShop); } catch (Exception) { return(null); } } Type nullable = Nullable.GetUnderlyingType(type); if (nullable != null) { //TODO:(Evgenii Babak) использовать Nameof() вместо названия типа string typeName = nullable.Name.ToLower(); switch (typeName) { case "int32": return(ToInt32Nullable(value)); case "int16": return(ToInt16Nullable(value)); case "datetime": return(ToDateTimeNullable(value)); case "bool": return(ToBoolNullable(value)); case "boolean": return(ToBoolNullable(value)); case "double": return(ToDoubleNullable(value)); default: return(null); } } else { string typeName = type.Name.ToLower(); switch (typeName) { case "string": return(ToString(value)); case "int32": return(ToInt32(value)); case "int16": return(ToInt16(value)); case "datetime": return(ToDateTime(value)); case "bool": return(ToBool(value)); case "boolean": return(ToBool(value)); case "byte[]": return(ToBytes(value)); case "double": return(ToDouble(value)); case "adtype": return((ADType)ToInt16(value)); case "averageutilization": return(ToAverageUtilization(value)); case "avionicsinventorymarktype": //case "atachapter": // return _casEnvironment.Dictionaries[typeof(AtaChapter)].GetItemById(ToInt32(value)); case "componentdirectivethreshold": return(ToComponentDirectiveThreshold(value)); case "trainingthreshold": return(ToTrainingThreshold(value)); case "componenttype": return(BaseComponentType.GetComponentTypeById(ToInt32(value))); case "componentrecordtype": return(ComponentRecordType.GetItemById(ToInt32(value))); case "detectionphase": return((DetectionPhase)ToInt16(value)); case "directivethreshold": return(ToDirectiveThreshold(value)); case "directivetype": return(DirectiveWorkType.GetItemById(ToInt32(value))); case "highlight": return(Highlight.GetHighlightById(ToInt32(value))); case "componentstatus": return((ComponentStatus)ToInt16(value)); case "lifelength": return(ToLifelength(value)); case "landinggearmarktype": return((LandingGearMarkType)ToInt32(value)); case "maintenancedirectivethreshold": return(ToMaintenanceDirectiveThreshold(value)); case "maintenancecontrolprocess": return(MaintenanceControlProcess.GetItemById(ToInt32(value))); case "powerloss": return((PowerLoss)ToInt16(value)); case "runupcondition": return((RunUpCondition)ToInt16(value)); case "runuptype": return((RunUpType)ToInt16(value)); case "shutdowntype": return((ShutDownType)ToInt16(value)); case "thrustlever": return((ThrustLever)ToInt16(value)); case "timespan": return(ToTime(value)); case "weathercondition": return((WeatherCondition)ToInt16(value)); case "workpackagestatus": return((WorkPackageStatus)ToInt32(value)); default: return(null); } } }
public override Task WriteResponseBodyAsync(OutputFormatterWriteContext context, Encoding selectedEncoding) { var query = (IQueryable)context.Object; var queryString = context.HttpContext.Request.QueryString; var columns = queryString.Value.Contains("$select") ? OutputFormatter.GetPropertiesFromSelect(queryString.Value, query.ElementType) : OutputFormatter.GetProperties(query.ElementType); var stream = new MemoryStream(); using (var document = SpreadsheetDocument.Create(stream, SpreadsheetDocumentType.Workbook)) { var workbookPart = document.AddWorkbookPart(); workbookPart.Workbook = new Workbook(); var worksheetPart = workbookPart.AddNewPart <WorksheetPart>(); worksheetPart.Worksheet = new Worksheet(); var workbookStylesPart = workbookPart.AddNewPart <WorkbookStylesPart>(); GenerateWorkbookStylesPartContent(workbookStylesPart); var sheets = workbookPart.Workbook.AppendChild(new Sheets()); var sheet = new Sheet() { Id = workbookPart.GetIdOfPart(worksheetPart), SheetId = 1, Name = "Sheet1" }; sheets.Append(sheet); workbookPart.Workbook.Save(); var sheetData = worksheetPart.Worksheet.AppendChild(new SheetData()); var headerRow = new Row(); foreach (var column in columns) { headerRow.Append(new Cell() { CellValue = new CellValue(column.Key), DataType = new EnumValue <CellValues>(CellValues.String) }); } sheetData.AppendChild(headerRow); foreach (var item in query) { var row = new Row(); foreach (var column in columns) { var value = OutputFormatter.GetValue(item, column.Key); var stringValue = $"{value}".Trim(); var cell = new Cell(); var underlyingType = column.Value.IsGenericType && column.Value.GetGenericTypeDefinition() == typeof(Nullable <>) ? Nullable.GetUnderlyingType(column.Value) : column.Value; var typeCode = Type.GetTypeCode(underlyingType); if (typeCode == TypeCode.DateTime) { if (stringValue != string.Empty) { cell.CellValue = new CellValue() { Text = DateTime.Parse(stringValue).ToOADate().ToString() }; cell.StyleIndex = (UInt32Value)1U; } } else if (typeCode == TypeCode.Boolean) { cell.CellValue = new CellValue(stringValue.ToLower()); cell.DataType = new EnumValue <CellValues>(CellValues.Boolean); } else if (IsNumeric(typeCode)) { cell.CellValue = new CellValue(stringValue); cell.DataType = new EnumValue <CellValues>(CellValues.Number); } else { cell.CellValue = new CellValue(stringValue); cell.DataType = new EnumValue <CellValues>(CellValues.String); } row.Append(cell); } sheetData.AppendChild(row); } workbookPart.Workbook.Save(); } if (stream?.Length > 0) { stream.Seek(0, SeekOrigin.Begin); } return(stream.CopyToAsync(context.HttpContext.Response.Body)); }
public static Type UnNullify(this Type type) { return(Nullable.GetUnderlyingType(type) ?? type); }
private bool ValidateRecursive(object obj, bool throwException, int deepLevel) { const int maxDeepLevel = 100; // Prevent "StackOverflowException" (this should never happen) if (++deepLevel == maxDeepLevel) { throw new InvalidOperationException("Recursive function call exceeded the permitted deep level."); } // Validate all properties with "CommandParameterAttribute" foreach (var property in CommandParameterAttribute.GetOrderedProperties(obj)) { // If property is value type and is not nullable, than must be set to nullable if (property.PropertyType.IsValueType && Nullable.GetUnderlyingType(property.PropertyType) == null) { if (throwException) { throw new InvalidOperationException($"'{property.Name}' property must be nullable."); } return(false); } } // Validate all properties with attributes derived from "ValidationAttribute" var results = new List <ValidationResult>(); Validator.TryValidateObject(obj, new ValidationContext(obj), results, true); if (results.Count > 0) { if (throwException) { throw new ArgumentException(results[0].ToString()); } return(false); } // Find all properties value with 'CommandBuilderObjectAttribute' and validate it recursively var properties = obj.GetType().GetProperties(BindingFlags.Public | BindingFlags.NonPublic | BindingFlags.Instance); foreach (var property in properties) { var propertyValue = property.GetValue(obj); if (propertyValue == null) { continue; } if (propertyValue.GetType().GetCustomAttribute <CommandBuilderObjectAttribute>() != null) { ValidateRecursive(propertyValue, throwException, deepLevel); } } return(true); }
public static DataTable ToDataTable <T>(List <T> items) { DataTable dataTable = new DataTable(typeof(T).Name); //Get all the properties PropertyInfo[] Props = typeof(T).GetProperties(BindingFlags.Public | BindingFlags.Instance); foreach (PropertyInfo prop in Props) { //Defining type of data column gives proper data table var type = (prop.PropertyType.IsGenericType && prop.PropertyType.GetGenericTypeDefinition() == typeof(Nullable <>) ? Nullable.GetUnderlyingType(prop.PropertyType) : prop.PropertyType); //Setting column names as Property names dataTable.Columns.Add(prop.Name, type); } foreach (T item in items) { var values = new object[Props.Length]; for (int i = 0; i < Props.Length; i++) { //inserting property values to datatable rows values[i] = Props[i].GetValue(item, null); } dataTable.Rows.Add(values); } //put a breakpoint here and check datatable return(dataTable); }
public static CompositeProperty AsCompositeProperty(this PdfAcroField o) { CompositeProperty _CompositeProperty = asCompositeProperty((dynamic)o); if (o.Flags == PdfAcroFieldFlags.Required) { if (_CompositeProperty.PropertyType != typeof(string)) { if (_CompositeProperty.PropertyType != typeof(byte[])) { _CompositeProperty.PropertyType = typeof(Nullable <>).MakeGenericType(Nullable.GetUnderlyingType(_CompositeProperty.PropertyType) ?? _CompositeProperty.PropertyType); } } } return(_CompositeProperty); }
public static DataTable ToDataTable <T>(IEnumerable <T> data, IEnumerable <PropertyInfo> properties) { var typeCasts = properties.Select(x => { var nullableUnderlyingType = Nullable.GetUnderlyingType(x.PropertyType); var type = nullableUnderlyingType ?? x.PropertyType; Type castType = null; if (type.IsEnum) { castType = Enum.GetUnderlyingType(type); } return(new { Name = x.Name, Nullable = nullableUnderlyingType != null, Type = castType ?? type, CastType = castType, Property = x }); } ).ToList(); var dataTable = new DataTable(); foreach (var item in typeCasts) { dataTable.Columns.Add( new DataColumn() { ColumnName = item.Name, AllowDBNull = item.Nullable, DataType = item.Type, } ); } foreach (var item in data) { dataTable.Rows.Add( typeCasts.Select(x => { var value = x.Property.GetValue(item, null); if (x.Nullable) { if (value == null) { return(DBNull.Value); } if (x.CastType != null) { return(Convert.ChangeType(value, x.CastType)); } return(Convert.ChangeType(value, x.Type)); } else { if (x.CastType != null) { return(Convert.ChangeType(value, x.CastType)); } return(value); } } ).ToArray() ); } return(dataTable); }
/// <summary> /// Adds or updates a value /// </summary> /// <param name="key">key to update</param> /// <param name="value">value to set</param> /// <returns> /// True if added or update and you need to save /// </returns> /// <exception cref="ArgumentException"></exception> public bool AddOrUpdateValue(string key, object value) { lock (locker) { Type typeOf = value.GetType(); if (typeOf.IsGenericType && typeOf.GetGenericTypeDefinition() == typeof(Nullable <>)) { typeOf = Nullable.GetUnderlyingType(typeOf); } var typeCode = Type.GetTypeCode(typeOf); switch (typeCode) { case TypeCode.Decimal: SharedPreferencesEditor.PutLong(key, (long)Convert.ToDecimal(value, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Boolean: SharedPreferencesEditor.PutBoolean(key, Convert.ToBoolean(value)); break; case TypeCode.Int64: SharedPreferencesEditor.PutLong(key, (long)Convert.ToInt64(value, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.String: SharedPreferencesEditor.PutString(key, Convert.ToString(value)); break; case TypeCode.Double: SharedPreferencesEditor.PutLong(key, (long)Convert.ToDouble(value, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Int32: SharedPreferencesEditor.PutInt(key, Convert.ToInt32(value, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Single: SharedPreferencesEditor.PutFloat(key, Convert.ToSingle(value, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.DateTime: SharedPreferencesEditor.PutLong(key, ((DateTime)(object)value).Ticks); break; default: if (value is Guid) { SharedPreferencesEditor.PutString(key, ((Guid)value).ToString()); } else { throw new ArgumentException(string.Format("Value of type {0} is not supported.", value.GetType().Name)); } break; } } lock (locker) { SharedPreferencesEditor.Commit(); } return(true); }
public TypePair GetAssociatedTypes(TypePair initialTypes) { return(new TypePair(Nullable.GetUnderlyingType(initialTypes.SourceType), initialTypes.DestinationType)); }
/// <summary> /// Gets the current value or the default that you specify. /// </summary> /// <typeparam name="T">Vaue of t (bool, int, float, long, string)</typeparam> /// <param name="key">Key for settings</param> /// <param name="defaultValue">default value if not set</param> /// <returns> /// Value or default /// </returns> /// <exception cref="ArgumentException"></exception> public T GetValueOrDefault <T>(string key, T defaultValue = default(T)) { lock (locker) { Type typeOf = typeof(T); if (typeOf.IsGenericType && typeOf.GetGenericTypeDefinition() == typeof(Nullable <>)) { typeOf = Nullable.GetUnderlyingType(typeOf); } object value = null; var typeCode = Type.GetTypeCode(typeOf); switch (typeCode) { case TypeCode.Decimal: value = (decimal)SharedPreferences.GetLong(key, (long)Convert.ToDecimal(defaultValue, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Boolean: value = SharedPreferences.GetBoolean(key, Convert.ToBoolean(defaultValue)); break; case TypeCode.Int64: value = (Int64)SharedPreferences.GetLong(key, (long)Convert.ToInt64(defaultValue, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.String: value = SharedPreferences.GetString(key, Convert.ToString(defaultValue)); break; case TypeCode.Double: value = (double)SharedPreferences.GetLong(key, (long)Convert.ToDouble(defaultValue, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Int32: value = SharedPreferences.GetInt(key, Convert.ToInt32(defaultValue, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.Single: value = SharedPreferences.GetFloat(key, Convert.ToSingle(defaultValue, System.Globalization.CultureInfo.InvariantCulture)); break; case TypeCode.DateTime: var ticks = SharedPreferences.GetLong(key, -1); if (ticks == -1) { value = defaultValue; } else { value = new DateTime(ticks); } break; default: if (defaultValue is Guid) { var outGuid = Guid.Empty; Guid.TryParse(SharedPreferences.GetString(key, Guid.Empty.ToString()), out outGuid); value = outGuid; } else { throw new ArgumentException(string.Format("Value of type {0} is not supported.", value.GetType().Name)); } break; } return(null != value ? (T)value : defaultValue); } }
public static object Aggregate <T>(this IQueryable <T> queryable, ICollection <Aggregator> aggregates) { if (aggregates == null || !aggregates.Any()) { return(null); } var objProps = new Dictionary <DynamicProperty, object>(); var groups = aggregates.GroupBy(g => g.Field); Type type; foreach (var group in groups) { var fieldProps = new Dictionary <DynamicProperty, object>(); foreach (var aggregate in @group) { var prop = typeof(T).GetProperty(aggregate.Field); var param = Expression.Parameter(typeof(T), "s"); var selector = aggregate.Aggregate == "count" && (Nullable.GetUnderlyingType(prop.PropertyType) != null) ? Expression.Lambda(Expression.NotEqual(Expression.MakeMemberAccess(param, prop), Expression.Constant(null, prop.PropertyType)), param) : Expression.Lambda(Expression.MakeMemberAccess(param, prop), param); var mi = aggregate.MethodInfo(typeof(T)); if (mi == null) { continue; } var val = queryable.Provider.Execute(Expression.Call(null, mi, aggregate.Aggregate == "count" && (Nullable.GetUnderlyingType(prop.PropertyType) == null) ? new[] { queryable.Expression } : new[] { queryable.Expression, Expression.Quote(selector) })); fieldProps.Add(new DynamicProperty(aggregate.Aggregate, typeof(object)), val); } type = DynamicExpression.CreateClass(fieldProps.Keys); var fieldObj = Activator.CreateInstance(type); foreach (var p in fieldProps.Keys) { type.GetProperty(p.Name).SetValue(fieldObj, fieldProps[p], null); } objProps.Add(new DynamicProperty(@group.Key, fieldObj.GetType()), fieldObj); } type = DynamicExpression.CreateClass(objProps.Keys); var obj = Activator.CreateInstance(type); foreach (var p in objProps.Keys) { type.GetProperty(p.Name).SetValue(obj, objProps[p], null); } return(obj); }
public static object GetPropertyValue(this object propertyValue, Type fieldType) { // Short-circuit conversion if the property value already if (fieldType.IsInstanceOfType(propertyValue)) { return(propertyValue); } if (fieldType.FullName == "System.Object") { return(propertyValue); } var enumerableInterface = fieldType.Name == "IEnumerable`1" ? fieldType : fieldType.GetInterface("IEnumerable`1"); if (fieldType.Name != "String" && enumerableInterface != null) { IList newArray; var elementType = enumerableInterface.GetGenericArguments()[0]; var underlyingType = Nullable.GetUnderlyingType(elementType) ?? elementType; var implementsIList = fieldType.GetInterface("IList") != null; if (implementsIList) { newArray = (IList)Activator.CreateInstance(fieldType); } else { var genericListType = typeof(List <>).MakeGenericType(elementType); newArray = (IList)Activator.CreateInstance(genericListType); } var valueList = propertyValue as IEnumerable; if (valueList == null) { return(newArray); } foreach (var listItem in valueList) { newArray.Add(listItem == null ? null : GetPropertyValue(listItem, underlyingType)); } return(newArray); } var value = propertyValue; var nullableFieldType = Nullable.GetUnderlyingType(fieldType); // if this is a nullable type and the value is null, return null if (nullableFieldType != null && value == null) { return(null); } if (nullableFieldType != null) { fieldType = nullableFieldType; } if (propertyValue is Dictionary <string, object> ) { return(ToObject(propertyValue as Dictionary <string, object>, fieldType)); } if (fieldType.GetTypeInfo().IsEnum) { if (value == null) { var enumNames = Enum.GetNames(fieldType); value = enumNames[0]; } if (!IsDefinedEnumValue(fieldType, value)) { throw new ExecutionError($"Unknown value '{value}' for enum '{fieldType.Name}'."); } var str = value.ToString(); value = Enum.Parse(fieldType, str, true); } return(ConvertValue(value, fieldType)); }