public void ListShards(IProjectionStore store) { var projections = store.Shards.Select(x => x.Source).Distinct(); if (projections.IsEmpty()) { AnsiConsole.Markup("[gray]No projections in this store.[/]"); AnsiConsole.WriteLine(); return; } var table = new Table(); table.AddColumn("Projection Name"); table.AddColumn("Class"); table.AddColumn("Shards"); table.AddColumn("Lifecycle"); foreach (var projection in projections) { var shards = store.Shards.Where(x => x.Source == projection).Select(x => x.Name.Identity).Join(", "); table.AddRow(projection.ProjectionName, projection.GetType().FullNameInCode(), shards, projection.Lifecycle.ToString()); } AnsiConsole.Render(table); AnsiConsole.WriteLine(); }
private int List(Settings settings) { var examples = _finder.FindExamples(); if (examples.Count == 0) { AnsiConsole.Markup("[yellow]No examples could be found.[/]"); return(0); } AnsiConsole.WriteLine(); var rows = new Grid().Collapse(); rows.AddColumn(); foreach (var group in examples.GroupBy(ex => ex.Group)) { rows.AddRow(CreateTable(settings, group.Key, group)); rows.AddEmptyRow(); } AnsiConsole.Render(rows); AnsiConsole.MarkupLine("Type [blue]dotnet example --help[/] for help"); return(0); }
public ProjectInformation FindExample(string name) { var result = new List <ProjectInformation>(); foreach (var example in FindExamples()) { if (example.Name.Equals(name, StringComparison.OrdinalIgnoreCase)) { result.Add(example); } } if (result.Count == 0) { AnsiConsole.Markup("[red]Error:[/] The example [underline]{0}[/] could not be found.", name); return(null); } if (result.Count > 1) { AnsiConsole.Markup("[red]Error:[/] Found multiple examples called [underline]{0}[/].", name); return(null); } return(result.First()); }
public override async Task <int> ExecuteAsync(CommandContext context, CheckSettings settings) { try { _pathToCsProjFile = _csProjFileService.GetPathToCsProjFile(settings.PathToCsProjFile); } catch (CsProjFileNotFoundException ex) { AnsiConsole.Markup($"[dim]WARN:[/] [red]{ex.Message}[/]"); return(await Task.FromResult(-1)); } AnsiConsole.MarkupLine($"Checking versions for [silver]{_pathToCsProjFile}[/]"); await _nuGetPackagesService.GetPackagesDataFromCsProjFileAsync(_pathToCsProjFile, _packages); if (_packages.Count == 0) { AnsiConsole.MarkupLine($"Could not find any packages in [silver]{_pathToCsProjFile}[/]"); return(await Task.FromResult(0)); } await _nuGetPackagesService.GetPackagesDataFromNugetRepositoryAsync(_pathToCsProjFile, _packages); PrintResult(); return(await Task.FromResult(0)); }
public void DisplayRebuildIsComplete() { AnsiConsole.Markup("[green]Projection Rebuild complete![/]"); AnsiConsole.WriteLine(); AnsiConsole.WriteLine(); AnsiConsole.WriteLine(); }
private void SaveGame() { AnsiConsole.Markup("[blue]Saving...[/]"); var json = JsonSerializer.Serialize(this.Game); File.WriteAllText(GameShell.SaveFile, json); AnsiConsole.MarkupLine("[green]Done![/]"); }
public async Task <int> InvokeAsync(InvocationContext context) { var directory = Directory.GetCurrentDirectory(); var bindingContextParseResult = context.BindingContext.ParseResult; var name = bindingContextParseResult.ValueForOption <string>("--name"); if (name == null) { AnsiConsole.Markup("[red]No project name specified[/]"); return(ExitCode.Error); } name = String.Join("_", name.Split(Path.GetInvalidFileNameChars(), StringSplitOptions.RemoveEmptyEntries)).TrimEnd('.'); var rootDir = Path.Combine(directory, name); if (Directory.Exists(rootDir)) { AnsiConsole.Markup($"[red]The folder {name} already exist. Please specify a new name.[/]"); return(ExitCode.Error); } Directory.CreateDirectory(rootDir); await File.WriteAllTextAsync(Path.Combine(rootDir, name + ".sln"), await GetReplacedTemplate(name, "sln_template")); var projectDir = Path.Combine(rootDir, name); Directory.CreateDirectory(projectDir); var propertiesDirectory = Path.Combine(projectDir, "Properties"); Directory.CreateDirectory(propertiesDirectory); var libProjectDir = Path.Combine(rootDir, name + ".lib"); Directory.CreateDirectory(libProjectDir); await File.WriteAllTextAsync(Path.Combine(projectDir, name + ".csproj"), await GetReplacedTemplate(name, "csproj_template")); await File.WriteAllTextAsync(Path.Combine(projectDir, name + ".csproj.user"), await GetReplacedTemplate(name, "csproj_user_template")); await File.WriteAllTextAsync(Path.Combine(libProjectDir, name + ".lib.csproj"), await GetReplacedTemplate(name, "lib_csproj_template")); await File.WriteAllTextAsync(Path.Combine(projectDir, "Test1.cs"), await GetReplacedTemplate(name, "test_template")); await File.WriteAllTextAsync(Path.Combine(projectDir, "Test2.cs"), await GetReplacedTemplate(name, "test2_template")); await File.WriteAllTextAsync(Path.Combine(projectDir, "Startup.cs"), await GetReplacedTemplate(name, "startup_template")); await File.WriteAllTextAsync(Path.Combine(projectDir, "appsettings.json"), await GetReplacedTemplate(name, "app_settings_template")); await File.WriteAllTextAsync(Path.Combine(propertiesDirectory, "launchSettings.json"), await GetReplacedTemplate(name, "launchSettings_template")); Console.WriteLine($"Created a new project with name: {name}"); return(ExitCode.Ok); }
private async Task ViewLeaderboard(int year, int board) { try { var leaders = await _mediator.Send(new LeaderboardQuery { Year = year, Board = board }); AnsiConsole.WriteLine(); AnsiConsoleHelper.TitleRule($":christmas_tree: Advent of Code Leaderboard {year}"); int place = 1; foreach (var member in leaders.Members.Values.OrderByDescending(m => m.LocalScore).ThenByDescending(m => m.Stars)) { AnsiConsole.Markup(string.Format("[white]{0,3}) {1,3}[/] ", place++, member.LocalScore)); for (int d = 1; d <= 25; d++) { if (DateTime.Now.Date < new DateTime(year, 12, d)) { AnsiConsole.Markup("[grey]·[/]"); continue; } string day = d.ToString(); string star; if (member.CompletionDayLevel.ContainsKey(day)) { Day completed = member.CompletionDayLevel[day]; if (completed.PartOneComplete && completed.PartTwoComplete) { star = "[yellow]*[/]"; } else { star = "[white]*[/]"; } } else { star = "[grey]*[/]"; } AnsiConsole.Markup(star); } AnsiConsole.MarkupLine($"[green] {member.Name}[/]"); } AnsiConsoleHelper.Rule("white"); } catch (UnconfiguredException ex) { AnsiConsole.MarkupLine($"[yellow][[:yellow_circle: {ex.Message}]][/]"); } }
public override async Task <int> ExecuteAsync(CommandContext context, UpgradeSettings settings) { // Get the path to the *.csproj file try { _pathToCsProjFile = _csProjFileService.GetPathToCsProjFile(settings.PathToCsProjFile); } catch (CsProjFileNotFoundException ex) { AnsiConsole.Markup($"[dim]WARN:[/] [red]{ex.Message}[/]"); return(await Task.FromResult(-1)); } AnsiConsole.MarkupLine($"Upgrading to [silver]{settings.Version}[/] versions in [silver]{_pathToCsProjFile}[/]"); // Check that packages are defined in the *.csproj file await _nuGetPackagesService.GetPackagesDataFromCsProjFileAsync(_pathToCsProjFile, _packages); if (_packages.Count == 0) { AnsiConsole.MarkupLine($"Could not find any packages in [silver]{_pathToCsProjFile}[/]"); return(await Task.FromResult(0)); } // If only a specific package should be upgraded, // ignore all the others if (!string.IsNullOrEmpty(settings.PackageToUpgrade)) { _packages.RemoveAll(p => p.PackageName != settings.PackageToUpgrade); } await _nuGetPackagesService.GetPackagesDataFromNugetRepositoryAsync(_pathToCsProjFile, _packages); await _csProjFileService.UpgradePackageVersionsAsync(_pathToCsProjFile, _packages, settings); if (!settings.DryRun) { if (settings.Interactive) { Console.WriteLine(); } AnsiConsole.MarkupLine( "[dim]INFO:[/] Run [blue]dotnet restore[/] to upgrade packages."); } Console.WriteLine(); return(await Task.FromResult(0)); }
/// <summary> /// Output the given text to the console in random ANSI colouring /// </summary> private static void SpanglyTitle(string title) { //var colours = new string[] { "red", "green", "yellow", "blue", "purple" }; var colours = new string[] { "red", "orange1", "yellow", "green", "blue", "purple", "violet" }; var rnd = new Random(); foreach (var c in $" * * * {title} * * * ") { var colour = colours[rnd.Next(colours.Length)]; AnsiConsole.Markup($"[bold {colour}]{c}[/]"); } AnsiConsole.WriteLine(); }
private async Task PrintResponse(HttpResponseMessage result) { var body = await result.Content.ReadAsStringAsync(); var headers = result.Headers.GetEnumerator(); var contentHeaders = result.Content.Headers.GetEnumerator(); if (_options.ShowHeader) { while (headers.MoveNext()) { var current = headers.Current; AnsiConsole.Markup($"[green]{current.Key}[/]: "); Console.WriteLine($"{String.Join(", ", current.Value)}"); } while (contentHeaders.MoveNext()) { var current = contentHeaders.Current; AnsiConsole.Markup($"[green]{current.Key}[/]: "); Console.WriteLine($"{String.Join(", ", current.Value)}"); } Console.WriteLine(); } if (_options.ShowStatus) { AnsiConsole.Markup($"[blue]{result.StatusCode}[/]\n"); Console.WriteLine(); } var ok = result.Content.Headers.TryGetValues("Content-Type", out var contentType); if (ok && contentType.Any(x => x.Contains("application/json"))) { var obj = JsonSerializer.Deserialize <dynamic>(body); var json = JsonSerializer.Serialize(obj, new JsonSerializerOptions { WriteIndented = true }); var pretty = UnescapeUnicode(json); Console.WriteLine(pretty); } else { Console.WriteLine(body); } }
static void Main(string[] args) { Dictionary <int, List <string> > answers = new Dictionary <int, List <string> >(); string filePath = AnsiConsole.Ask <string>("Please Enter the file path."); InputReader inputReader = new InputReader(filePath); //var survey = new Survey(); //var question = survey.GetQuestion(); var question = inputReader.GetQuestion(); var prompt = new TextPrompt <string>(question.Question); foreach (var a in question.Answers) { prompt.AddChoice(a); } var answer = AnsiConsole.Prompt(prompt); List <string> lst_answ = new List <string>(); lst_answ.Add(question.Question); lst_answ.Add(answer); answers.Add(1, lst_answ); Console.WriteLine(answers); if (answer == inputReader.GetCorrectAnswer()) { AnsiConsole.Markup("That's [underline green]correct[/]!"); } // var prompt = new TextPrompt<string>(question.Question); // foreach(var a in question.Answers) { // prompt.AddChoice(a); // } // var answer = AnsiConsole.Prompt(prompt); // if(answer == survey.GetCorrectAnswer()) { // AnsiConsole.Markup("That's [underline green]correct[/]!"); // } // else { // AnsiConsole.Markup("That's [underline red]wrong[/]!"); // } }
private void LoadExistingWorld() { if (File.Exists(GameShell.SaveFile)) { AnsiConsole.Markup("[blue]Loading...[/]"); var json = File.ReadAllText(GameShell.SaveFile); this.Game = JsonSerializer.Deserialize <Game>(json); AnsiConsole.MarkupLine("[green]Done![/]"); this.GameLoop(); } else { AnsiConsole.MarkupLine("[red]A save file does not exist.[/]"); } }
private int List() { var examples = _finder.FindExamples(); if (examples.Count == 0) { AnsiConsole.Markup("[yellow]No examples could be found.[/]"); return(0); } var grid = new Table { Border = TableBorder.Rounded }; grid.AddColumn(new TableColumn("[grey]Name[/]") { NoWrap = true, }); grid.AddColumn(new TableColumn("[grey]Path[/]") { NoWrap = true, }); grid.AddColumn(new TableColumn("[grey]Description[/]")); foreach (var example in examples.OrderBy(e => e.Order)) { var path = _environment.WorkingDirectory.GetRelativePath(example.Path); grid.AddRow( $"[underline blue]{example.Name}[/]", $"[grey]{path.FullPath}[/]", !string.IsNullOrEmpty(example.Description) ? $"{example.Description}" : "[grey]N/A[/]"); } AnsiConsole.WriteLine(); AnsiConsole.MarkupLine("[u]Available examples:[/]"); AnsiConsole.WriteLine(); AnsiConsole.Render(grid); AnsiConsole.WriteLine(); AnsiConsole.MarkupLine("Type [blue]dotnet example --help[/] for help"); return(0); }
private static void RunConsole() { try { AnsiConsole.Render(new FigletText("PhotoSync").Centered()); AnsiConsole.Render(new Rule()); AnsiConsole.Markup("[underline red]Hello[/] World!"); } catch (Exception ex) { Console.WriteLine("Error occurred:"); Console.WriteLine(ex.Message); } finally { Console.WriteLine("Press any key to exit."); _ = Console.ReadKey(); } }
private FilePath?FindProgram(ProjectInformation project) { var directory = project.Path.GetDirectory(); var result = _globber.Match("**/Program.{f|c}s", new GlobberSettings { Root = directory }).OfType <FilePath>().ToList(); if (result.Count == 0) { AnsiConsole.Markup("[red]Error:[/] Could not find Program.cs for example [underline]{0}[/].", project.Name); return(null); } if (result.Count > 1) { AnsiConsole.Markup("[red]Error:[/] Found multiple Program.cs for example [underline]{0}[/].", project.Name); return(null); } return(result.First()); }
public async ValueTask ExecuteAsync(IConsole console) { var(draftFileInfo, draftFrontMatter) = await BlogUtils.AskUserToSelectDraft("Which draft do you want to publish?"); var blogPostPath = Path.Combine(BlogSettings.PostsFolder, draftFileInfo.Name); if (!Overwrite && File.Exists(blogPostPath)) { await console.Output.WriteLineAsync($"File exists, please use {nameof(Overwrite)} parameter."); return; } var updatedDraftLines = await GetUpdatedDraftFrontMatterLines(draftFileInfo.FullName, draftFrontMatter); await File.WriteAllLinesAsync(blogPostPath, updatedDraftLines); File.Delete(draftFileInfo.FullName); AnsiConsole.Markup($"Published [green]{blogPostPath}[/]"); }
public async Task <int> InvokeAsync(InvocationContext context) { var result = context.BindingContext.ParseResult; var fileInfo = result.ValueForOption <FileInfo>("--file"); if (fileInfo == null) { AnsiConsole.Markup("[red]Could not find the specified file[/]"); return(ExitCode.Error); } var debugEnabled = result.ValueForOption <bool>("--debug"); try { var additionalArgs = new List <string>(); if (debugEnabled) { additionalArgs.Add("--debug"); } //Always report stats back to main process additionalArgs.Add("--out"); additionalArgs.Add("pipe"); var resultOfRun = await _runEngine.Execute(fileInfo, additionalArgs, _hostApplicationLifetime.ApplicationStopping); if (resultOfRun) { return(ExitCode.Ok); } return(ExitCode.Error); } catch (Exception e) { AnsiConsole.WriteException(e); return(ExitCode.Error); } }
static void Main(string[] args) { var survey = new Survey(); var question = survey.GetQuestion(); var prompt = new TextPrompt <string>(question.Question); foreach (var a in question.Answers) { prompt.AddChoice(a); } var answer = AnsiConsole.Prompt(prompt); if (answer == survey.GetCorrectAnswer()) { AnsiConsole.Markup("That's [underline green]correct[/]!"); } else { AnsiConsole.Markup("That's [underline red]wrong[/]!"); } }
public async ValueTask ExecuteAsync(IConsole console) { var now = DateTime.Now; var slug = Title.ToUrlSlug(); var stringBuilder = new StringBuilder(); stringBuilder.AppendLine("---"); stringBuilder.AppendLine($"title: '{Title}'"); stringBuilder.AppendLine(now.ToPermalink(slug)); stringBuilder.AppendLine($"date: {now}"); stringBuilder.AppendLine(now.ToDisqusIdentifier()); stringBuilder.AppendLine("coverSize: partial"); stringBuilder.AppendLine("tags: [ASP.NET Core, Microsoft Azure, Docker]"); stringBuilder.AppendLine("coverCaption: 'LO Ferré, Petite Anse, Martinique, France'"); stringBuilder.AppendLine("coverImage: 'https://c7.staticflickr.com/9/8689/16775792438_e45283970c_h.jpg'"); stringBuilder.AppendLine("thumbnailImage: 'https://c7.staticflickr.com/9/8689/16775792438_8366ee5732_q.jpg'"); stringBuilder.AppendLine("---"); stringBuilder.AppendLine("Text displayed on the home page"); stringBuilder.AppendLine("<!-- more -->"); stringBuilder.AppendLine("Continue with text displayed on the blog page"); stringBuilder.AppendLine("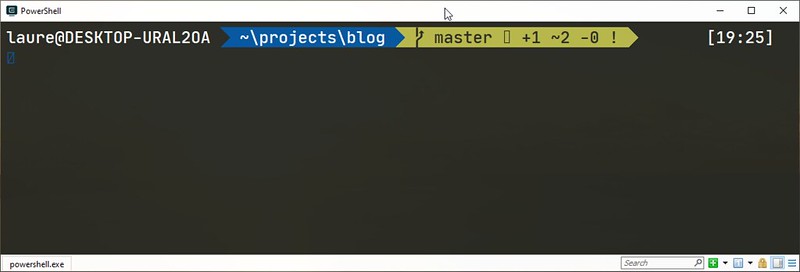"); stringBuilder.AppendLine("{% alert info %}"); stringBuilder.AppendLine("{% endalert %}"); stringBuilder.AppendLine("{% codeblock GreeterService.cs lang:csharp %}"); stringBuilder.AppendLine("{% endcodeblock %}"); stringBuilder.AppendLine("# Conclusion"); stringBuilder.AppendLine("TODO"); stringBuilder.AppendLine("<p></p>"); stringBuilder.AppendLine("{% githubCard user:laurentkempe repo:dotfiles align:left %}"); var filename = $"{slug}.md"; var path = Path.Combine(BlogSettings.DraftsFolder, filename); await File.WriteAllTextAsync(path, stringBuilder.ToString()); AnsiConsole.Markup($"Successfully created [green]{filename}[/]"); }
public override async Task <int> ExecuteAsync(CommandContext context, CheckSettings settings) { Util.Verbose = settings.Verbose; Util.CI = settings.CI; if (settings.CI) { settings.NonInteractive = true; } Console.Title = ToolInfo.ToolName; AnsiConsole.Render( new FigletText(".NET MAUI").LeftAligned().Color(Color.Green)); AnsiConsole.MarkupLine($"[underline bold green]{Icon.Ambulance} {ToolInfo.ToolName} {Icon.Recommend}[/]"); AnsiConsole.Render(new Rule()); AnsiConsole.MarkupLine("This tool will attempt to evaluate your .NET MAUI development environment."); AnsiConsole.MarkupLine("If problems are detected, this tool may offer the option to try and fix them for you, or suggest a way to fix them yourself."); AnsiConsole.WriteLine(); AnsiConsole.MarkupLine("Thanks for choosing .NET MAUI!"); AnsiConsole.Render(new Rule()); if (!Util.IsAdmin() && Util.IsWindows) { var suTxt = Util.IsWindows ? "Administrator" : "Superuser (su)"; AnsiConsole.MarkupLine($"[bold red]{Icon.Bell} {suTxt} is required to fix most issues. Consider exiting and running the tool with {suTxt} permissions.[/]"); AnsiConsole.Render(new Rule()); if (!settings.NonInteractive) { if (!AnsiConsole.Confirm("Would you still like to continue?", false)) { return(1); } } } var cts = new System.Threading.CancellationTokenSource(); var checkupStatus = new Dictionary <string, Models.Status>(); var sharedState = new SharedState(); var results = new Dictionary <string, DiagnosticResult>(); var consoleStatus = AnsiConsole.Status(); var skippedChecks = new List <string>(); AnsiConsole.Markup($"[bold blue]{Icon.Thinking} Synchronizing configuration...[/]"); var manifest = await ToolInfo.LoadManifest(settings.Manifest, settings.Dev); if (!ToolInfo.Validate(manifest)) { ToolInfo.ExitPrompt(settings.NonInteractive); return(-1); } AnsiConsole.MarkupLine(" ok"); AnsiConsole.Markup($"[bold blue]{Icon.Thinking} Scheduling appointments...[/]"); if (!string.IsNullOrEmpty(settings.DotNetSdkRoot)) { sharedState.SetEnvironmentVariable("DOTNET_ROOT", settings.DotNetSdkRoot); } var checkups = CheckupManager.BuildCheckupGraph(manifest, sharedState); AnsiConsole.MarkupLine(" ok"); var checkupId = string.Empty; for (int i = 0; i < checkups.Count(); i++) { var checkup = checkups.ElementAt(i); // Set the manifest checkup.Manifest = manifest; // If the ID is the same, it's a retry var isRetry = checkupId == checkup.Id; // Track the last used id so we can detect retry checkupId = checkup.Id; if (!checkup.ShouldExamine(sharedState)) { checkupStatus[checkup.Id] = Models.Status.Ok; continue; } var skipCheckup = false; var dependencies = checkup.DeclareDependencies(checkups.Select(c => c.Id)); // Make sure our dependencies succeeded first if (dependencies?.Any() ?? false) { foreach (var dep in dependencies) { var depCheckup = checkups.FirstOrDefault(c => c.Id.StartsWith(dep.CheckupId, StringComparison.OrdinalIgnoreCase)); if (depCheckup != null && depCheckup.IsPlatformSupported(Util.Platform)) { if (!checkupStatus.TryGetValue(dep.CheckupId, out var depStatus) || depStatus == Models.Status.Error) { skipCheckup = dep.IsRequired; break; } } } } // See if --skip was specified if (settings.Skip?.Any(s => s.Equals(checkup.Id, StringComparison.OrdinalIgnoreCase) || s.Equals(checkup.GetType().Name, StringComparison.OrdinalIgnoreCase)) ?? false) { skipCheckup = true; } if (skipCheckup) { skippedChecks.Add(checkup.Id); checkupStatus[checkup.Id] = Models.Status.Error; AnsiConsole.WriteLine(); AnsiConsole.MarkupLine($"[bold red]{Icon.Error} Skipped: " + checkup.Title + "[/]"); continue; } checkup.OnStatusUpdated += checkupStatusUpdated; AnsiConsole.WriteLine(); AnsiConsole.MarkupLine($"[bold]{Icon.Checking} " + checkup.Title + " Checkup[/]..."); Console.Title = checkup.Title; DiagnosticResult diagnosis = null; try { diagnosis = await checkup.Examine(sharedState); } catch (Exception ex) { Util.Exception(ex); diagnosis = new DiagnosticResult(Models.Status.Error, checkup, ex.Message); } results[checkup.Id] = diagnosis; // Cache the status for dependencies checkupStatus[checkup.Id] = diagnosis.Status; if (diagnosis.Status == Models.Status.Ok) { continue; } var statusEmoji = diagnosis.Status == Models.Status.Error ? Icon.Error : Icon.Warning; var statusColor = diagnosis.Status == Models.Status.Error ? "red" : "darkorange3_1"; var msg = !string.IsNullOrEmpty(diagnosis.Message) ? " - " + diagnosis.Message : string.Empty; if (diagnosis.HasSuggestion) { Console.WriteLine(); AnsiConsole.Render(new Rule()); AnsiConsole.MarkupLine($"[bold blue]{Icon.Recommend} Recommendation:[/][blue] {diagnosis.Suggestion.Name}[/]"); if (!string.IsNullOrEmpty(diagnosis.Suggestion.Description)) { AnsiConsole.MarkupLine("" + diagnosis.Suggestion.Description + ""); } AnsiConsole.Render(new Rule()); Console.WriteLine(); // See if we should fix // needs to have a remedy available to even bother asking/trying var doFix = diagnosis.Suggestion.HasSolution && ( // --fix + --non-interactive == auto fix, no prompt (settings.NonInteractive && settings.Fix) // interactive (default) + prompt/confirm they want to fix || (!settings.NonInteractive && AnsiConsole.Confirm($"[bold]{Icon.Bell} Attempt to fix?[/]")) ); if (doFix && !isRetry) { var isAdmin = Util.IsAdmin(); var adminMsg = Util.IsWindows ? $"{Icon.Bell} [red]Administrator Permissions Required. Try opening a new console as Administrator and running this tool again.[/]" : $"{Icon.Bell} [red]Super User Permissions Required. Try running this tool again with 'sudo'.[/]"; var didFix = false; foreach (var remedy in diagnosis.Suggestion.Solutions) { try { remedy.OnStatusUpdated += remedyStatusUpdated; AnsiConsole.MarkupLine($"{Icon.Thinking} Attempting to fix: " + checkup.Title); await remedy.Implement(sharedState, cts.Token); didFix = true; AnsiConsole.MarkupLine($"[bold]Fix applied. Checking again...[/]"); } catch (Exception x) when(x is AccessViolationException || x is UnauthorizedAccessException) { Util.Exception(x); AnsiConsole.Markup(adminMsg); } catch (Exception ex) { Util.Exception(ex); AnsiConsole.MarkupLine("[bold red]Fix failed - " + ex.Message + "[/]"); } finally { remedy.OnStatusUpdated -= remedyStatusUpdated; } } // RETRY The check again if (didFix) { i--; } } } checkup.OnStatusUpdated -= checkupStatusUpdated; } AnsiConsole.Render(new Rule()); AnsiConsole.WriteLine(); var erroredChecks = results.Values.Where(d => d.Status == Models.Status.Error && !skippedChecks.Contains(d.Checkup.Id)); foreach (var ec in erroredChecks) { Util.Log($"Checkup had Error status: {ec.Checkup.Id}"); } var hasErrors = erroredChecks.Any(); if (hasErrors) { AnsiConsole.MarkupLine($"[bold red]{Icon.Bell} There were one or more problems detected.[/]"); AnsiConsole.MarkupLine($"[bold red]Please review the errors and correct them and run {ToolInfo.ToolCommand} again.[/]"); } else { AnsiConsole.MarkupLine($"[bold blue]{Icon.Success} Congratulations, everything looks great![/]"); } Console.Title = ToolInfo.ToolName; ToolInfo.ExitPrompt(settings.NonInteractive); Util.Log($"Has Errors? {hasErrors}"); var exitCode = hasErrors ? 1 : 0; Environment.ExitCode = exitCode; return(exitCode); }
public void DisplayNoStoresMessage() { AnsiConsole.Markup("[gray]No document stores in this application.[/]"); }
public void DisplayNoAsyncProjections() { AnsiConsole.Markup("[gray]No asynchronous projections match the criteria.[/]"); AnsiConsole.WriteLine(); }
public void DisplayNoDatabases() { AnsiConsole.Markup("[gray]No named databases match the criteria.[/]"); AnsiConsole.WriteLine(); }
public void DisplayNoMatchingProjections() { AnsiConsole.Markup("[gray]No projections match the criteria.[/]"); AnsiConsole.WriteLine(); }
public override async Task <int> ExecuteAsync(CommandContext context, DoctorSettings settings) { Console.Title = ToolName; AnsiConsole.MarkupLine($"[underline bold green]{Icon.Ambulance} {ToolName} {Icon.Recommend}[/]"); AnsiConsole.Render(new Rule()); AnsiConsole.MarkupLine("This tool will attempt to evaluate your .NET MAUI development environment."); AnsiConsole.MarkupLine("If problems are detected, this tool may offer the option to try and fix them for you, or suggest a way to fix them yourself."); AnsiConsole.WriteLine(); AnsiConsole.MarkupLine("Thanks for choosing .NET MAUI!"); AnsiConsole.WriteLine(); if (!settings.NonInteractive) { AnsiConsole.Markup("Press any key to start..."); Console.ReadKey(); AnsiConsole.WriteLine(); } AnsiConsole.Render(new Rule()); var clinic = new Clinic(); var cts = new System.Threading.CancellationTokenSource(); var checkupStatus = new Dictionary <string, Doctoring.Status>(); var patientHistory = new PatientHistory(); var diagnoses = new List <Diagonosis>(); var consoleStatus = AnsiConsole.Status(); AnsiConsole.Markup($"[bold blue]{Icon.Thinking} Synchronizing configuration...[/]"); var chart = await Manifest.Chart.FromFileOrUrl(settings.Manifest); var toolVersion = chart?.Doctor?.ToolVersion; var fileVersion = NuGetVersion.Parse(FileVersionInfo.GetVersionInfo(this.GetType().Assembly.Location).FileVersion); if (string.IsNullOrEmpty(toolVersion) || !NuGetVersion.TryParse(toolVersion, out var toolVer) || fileVersion < toolVer) { Console.WriteLine(); AnsiConsole.MarkupLine($"[bold red]{Icon.Error} Updating to version {toolVersion} or newer is required:[/]"); AnsiConsole.MarkupLine($"[red]Update with the following:[/]"); var installCmdVer = string.IsNullOrEmpty(toolVersion) ? "" : $" --version {toolVersion}"; AnsiConsole.Markup($" dotnet tool install --global {ToolPackageId}{installCmdVer}"); return(-1); } AnsiConsole.MarkupLine(" ok"); AnsiConsole.Markup($"[bold blue]{Icon.Thinking} Scheduling appointments...[/]"); if (chart.Doctor.OpenJdk != null) { clinic.OfferService(new OpenJdkCheckup(chart.Doctor.OpenJdk.MinimumVersion, chart.Doctor.OpenJdk.ExactVersion)); } if (chart.Doctor.Android != null) { clinic.OfferService(new AndroidSdkManagerCheckup()); clinic.OfferService(new AndroidSdkPackagesCheckup(chart.Doctor.Android.Packages.ToArray())); clinic.OfferService(new AndroidSdkLicensesCheckup()); } if (chart.Doctor.XCode != null) { clinic.OfferService(new XCodeCheckup(chart.Doctor.XCode.MinimumVersion, chart.Doctor.XCode.ExactVersion)); } if (chart.Doctor.VSMac != null && !string.IsNullOrEmpty(chart.Doctor.VSMac.MinimumVersion)) { clinic.OfferService(new VisualStudioMacCheckup(chart.Doctor.VSMac.MinimumVersion, chart.Doctor.VSMac.ExactVersion)); } if (chart.Doctor.VSWin != null && !string.IsNullOrEmpty(chart.Doctor.VSWin.MinimumVersion)) { clinic.OfferService(new VisualStudioWindowsCheckup(chart.Doctor.VSWin.MinimumVersion, chart.Doctor.VSWin.ExactVersion)); } if (chart.Doctor.DotNet?.Sdks?.Any() ?? false) { clinic.OfferService(new DotNetCheckup(chart.Doctor.DotNet.Sdks.ToArray())); foreach (var sdk in chart.Doctor.DotNet.Sdks) { clinic.OfferService(new DotNetWorkloadsCheckup(sdk.Version, sdk.Workloads.ToArray(), sdk.PackageSources.ToArray())); // Always run the packs checkup even if manifest is empty, since the workloads may resolve some required packs dynamically that aren't from the manifest clinic.OfferService(new DotNetPacksCheckup(sdk.Version, sdk.Packs?.ToArray() ?? Array.Empty <Manifest.NuGetPackage>(), sdk.PackageSources.ToArray())); } } var checkups = clinic.ScheduleCheckups(); AnsiConsole.MarkupLine(" ok"); foreach (var checkup in checkups) { var skipCheckup = false; // Make sure our dependencies succeeded first if (checkup.Dependencies?.Any() ?? false) { foreach (var dep in checkup.Dependencies) { if (!checkupStatus.TryGetValue(dep.CheckupId, out var depStatus) || depStatus != Doctoring.Status.Ok) { skipCheckup = dep.IsRequired; break; } } } if (skipCheckup) { checkupStatus.Add(checkup.Id, Doctoring.Status.Error); AnsiConsole.WriteLine(); AnsiConsole.MarkupLine($"[bold red]{Icon.Error} Skipped: " + checkup.Title + "[/]"); continue; } checkup.OnStatusUpdated += (s, e) => { var msg = ""; if (e.Status == Doctoring.Status.Error) { msg = $"[red]{Icon.Error} {e.Message}[/]"; } else if (e.Status == Doctoring.Status.Warning) { msg = $"[red]{Icon.Error} {e.Message}[/]"; } else if (e.Status == Doctoring.Status.Ok) { msg = $"[green]{Icon.Success} {e.Message}[/]"; } else { msg = $"{Icon.ListItem} {e.Message}"; } AnsiConsole.MarkupLine(" " + msg); }; AnsiConsole.WriteLine(); AnsiConsole.MarkupLine($"[bold]{Icon.Checking} " + checkup.Title + " Checkup[/]..."); Console.Title = checkup.Title; Diagonosis diagnosis = null; try { diagnosis = await checkup.Examine(patientHistory); } catch (Exception ex) { diagnosis = new Diagonosis(Doctoring.Status.Error, checkup, ex.Message); } diagnoses.Add(diagnosis); // Cache the status for dependencies checkupStatus.Add(checkup.Id, diagnosis.Status); if (diagnosis.Status == Doctoring.Status.Ok) { continue; } var statusEmoji = diagnosis.Status == Doctoring.Status.Error ? Icon.Error : Icon.Warning; var statusColor = diagnosis.Status == Doctoring.Status.Error ? "red" : "orange3"; var msg = !string.IsNullOrEmpty(diagnosis.Message) ? " - " + diagnosis.Message : string.Empty; //AnsiConsole.MarkupLine($"[{statusColor}]{statusEmoji} {checkup.Title}{msg}[/]"); if (diagnosis.HasPrescription) { AnsiConsole.MarkupLine($" [bold blue]{Icon.Recommend} Recommendation:[/] {diagnosis.Prescription.Name}"); if (!string.IsNullOrEmpty(diagnosis.Prescription.Description)) { AnsiConsole.MarkupLine(" " + diagnosis.Prescription.Description); } // See if we should fix // needs to have a remedy available to even bother asking/trying var doFix = diagnosis.Prescription.HasRemedy && ( // --fix + --non-interactive == auto fix, no prompt (settings.NonInteractive && settings.Fix) // interactive (default) + prompt/confirm they want to fix || (!settings.NonInteractive && AnsiConsole.Confirm($" [bold]{Icon.Bell} Attempt to fix?[/]")) ); if (doFix) { var isAdmin = Util.IsAdmin(); foreach (var remedy in diagnosis.Prescription.Remedies) { if (!remedy.HasPrivilegesToRun(isAdmin, Util.Platform)) { AnsiConsole.Markup("Fix requires running with adminstrator privileges. Try opening a terminal as administrator and running maui-doctor again."); continue; } try { remedy.OnStatusUpdated += (s, e) => { AnsiConsole.MarkupLine(" " + e.Message); }; AnsiConsole.MarkupLine($"{Icon.Thinking} Attempting to fix: " + checkup.Title); await remedy.Cure(cts.Token); AnsiConsole.MarkupLine(" Fix applied. Run doctor again to verify."); } catch (Exception ex) { AnsiConsole.MarkupLine(" Fix failed - " + ex.Message); } } } } } AnsiConsole.Render(new Rule()); AnsiConsole.WriteLine(); if (diagnoses.Any(d => d.Status == Doctoring.Status.Error)) { AnsiConsole.MarkupLine($"[bold red]{Icon.Bell} There were one or more problems detected.[/]"); AnsiConsole.MarkupLine($"[bold red]Please review the errors and correct them and run maui-doctor again.[/]"); } else { AnsiConsole.MarkupLine($"[bold blue]{Icon.Success} Congratulations, everything looks great![/]"); } Console.Title = ToolName; return(0); }
public void DisplayEmptyEventsMessage(IProjectionStore store) { AnsiConsole.Markup("[bold]The event storage is empty, aborting.[/]"); }
public override async Task <int> ExecuteAsync(CommandContext context, ConfigSettings settings) { AnsiConsole.Markup($"[bold blue]{Icon.Thinking} Synchronizing configuration...[/]"); var manifest = await ToolInfo.LoadManifest(settings.Manifest, settings.Dev); if (!ToolInfo.Validate(manifest)) { ToolInfo.ExitPrompt(settings.NonInteractive); return(-1); } AnsiConsole.MarkupLine(" ok"); var manifestDotNetSdk = manifest?.Check?.DotNet?.Sdks?.FirstOrDefault(); var manifestNuGetSources = manifestDotNetSdk?.PackageSources; if (manifestDotNetSdk != null && (settings.DotNetAllowPrerelease.HasValue || !string.IsNullOrEmpty(settings.DotNetRollForward) || settings.DotNetVersion)) { // Write global.json or update global.json /* * * { * "sdk": { * "version" : "", * "allowPrerelease": true, * "rollForward": "patch|feature|minor|major|latestPatch|latestFeature|latestMinor|latestMajor|disable" * } * } */ const string globalJsonFile = "global.json"; DotNetGlobalJson globaljson = default; if (File.Exists(globalJsonFile)) { try { globaljson = JsonConvert.DeserializeObject <DotNetGlobalJson>(File.ReadAllText(globalJsonFile)); } catch { } } if (globaljson == null) { globaljson = new DotNetGlobalJson(); } if (settings.DotNetVersion) { Util.Log($"Setting version in global.json: {manifestDotNetSdk.Version}"); globaljson.Sdk.Version = manifestDotNetSdk.Version; AnsiConsole.MarkupLine($"[green]{Icon.Success} Set global.json 'version': {manifestDotNetSdk.Version}[/]"); } if (settings?.DotNetAllowPrerelease.HasValue ?? false) { Util.Log($"Setting allowPrerelease in global.json: {settings.DotNetAllowPrerelease.Value}"); globaljson.Sdk.AllowPrerelease = settings.DotNetAllowPrerelease.Value; AnsiConsole.MarkupLine($"[green]{Icon.Success} Set global.json 'allowPrerelease': {settings.DotNetAllowPrerelease.Value}[/]"); } if (!string.IsNullOrEmpty(settings.DotNetRollForward)) { if (!DotNetGlobalJsonSdk.ValidRollForwardValues.Contains(settings.DotNetRollForward)) { throw new ArgumentException("Invalid rollForward value specified. Must be one of: ", string.Join(", ", DotNetGlobalJsonSdk.ValidRollForwardValues)); } Util.Log($"Setting rollForward in global.json: {settings.DotNetRollForward}"); globaljson.Sdk.RollForward = settings.DotNetRollForward; AnsiConsole.MarkupLine($"[green]{Icon.Success} Set global.json 'rollForward': {settings.DotNetRollForward}[/]"); } File.WriteAllText(globalJsonFile, JsonConvert.SerializeObject(globaljson, Formatting.Indented)); } if ((manifestNuGetSources?.Any() ?? false) && settings.NuGetSources) { // write nuget.config or update var nugetConfigFile = "NuGet.config"; var nugetRoot = Directory.GetCurrentDirectory(); ISettings ns = new Settings(nugetRoot, nugetConfigFile); if (File.Exists(Path.Combine(nugetRoot, nugetConfigFile))) { try { ns = Settings.LoadSpecificSettings(nugetRoot, nugetConfigFile); } catch { } } var packageSourceProvider = new PackageSourceProvider(ns); var addedAny = false; foreach (var src in manifestNuGetSources) { var srcExists = false; try { var existingSrc = packageSourceProvider.GetPackageSourceBySource(src); if (existingSrc != null) { srcExists = true; } } catch { } if (srcExists) { Util.Log($"PackageSource already exists in NuGet.config: {src}"); AnsiConsole.MarkupLine($"{Icon.ListItem} PackageSource exists in NuGet.config: {src}"); } else { Util.Log($"Adding PackageSource to NuGet.config: {src}"); packageSourceProvider.AddPackageSource(new PackageSource(src)); addedAny = true; AnsiConsole.MarkupLine($"[green]{Icon.Success} Added PackageSource to NuGet.config: {src}[/]"); } } if (addedAny) { Util.Log($"Saving NuGet.config"); ns.SaveToDisk(); } } ToolInfo.ExitPrompt(settings.NonInteractive); return(0); }
static void Main(string[] args) { GradientMarkup.DefaultStartColor = "#ed4264"; GradientMarkup.DefaultEndColor = "#ffedbc"; AnsiConsole.Markup(GradientMarkup.Ascii("Awesome Miner", FigletFont.Default, "#009245", "#FCEE21")); AnsiConsole.Markup(GradientMarkup.Text("Awesome Miner v8.4.x Keygen by Ankur Mathur")); var filePath = Path.Combine(Environment.CurrentDirectory, "AwesomeMiner.exe"); if (!File.Exists(filePath)) { AnsiConsole.MarkupLine($"\r\n[bold red]File not found in the current directory ![/] ({filePath})"); Console.ReadKey(); Environment.Exit(0); } var p = new Patcher(filePath); var licMgr = p.FindMethodsByOpCodeSignature( OpCodes.Call, OpCodes.Ldarg_1, OpCodes.Stfld); foreach (var item in licMgr) { var ins = p.GetInstructions(item); var operands = ins.Where(x => x.OpCode == OpCodes.Stfld).Select(x => x.Operand).OfType <dnlib.DotNet.FieldDef>(); if (operands.Any(x => x.Name == "RegistrationSettings")) { item.Method = null; CLS_LicenseManager = item; AnsiConsole.Markup(GradientMarkup.Text($"Found LicenseManager Class at : {CLS_LicenseManager.Class}")); break; } } var targets = p.FindMethodsByOpCodeSignature( OpCodes.Ldc_I4_1, OpCodes.Newarr, OpCodes.Stloc_0, OpCodes.Ldloc_0, OpCodes.Ldc_I4_0, OpCodes.Ldarg_0, OpCodes.Stelem_Ref, OpCodes.Call, OpCodes.Call, OpCodes.Ldstr, OpCodes.Ldloc_0, OpCodes.Call, OpCodes.Unbox, OpCodes.Ldobj, OpCodes.Ret).Where(t => t.Class.Equals(CLS_LicenseManager.Class)).ToArray(); FN_GetSoftwareEdition = targets.FirstOrDefault(x => p.FindInstruction(x, Instruction.Create(OpCodes.Unbox, new TypeDefUser("AwesomeMiner.Components.SoftwareEdition"))) > -1); if (FN_GetSoftwareEdition != null) { AnsiConsole.Markup(GradientMarkup.Text($"GetSoftwareEdition Method at : {FN_GetSoftwareEdition.Method} ... ")); FN_GetSoftwareEdition.Instructions = new Instruction[] { Instruction.Create(OpCodes.Ldc_I4, 10), Instruction.Create(OpCodes.Ret) }; p.Patch(FN_GetSoftwareEdition); AnsiConsole.Markup("[Green]Success[/]"); } targets = p.FindMethodsByArgumentSignatureExact(CLS_LicenseManager, null, "AwesomeMiner.Entities.Settings.RegistrationSettings", "System.Boolean", "System.String"); FN_ValidateRegistrationCode = targets.FirstOrDefault(); if (FN_ValidateRegistrationCode != null) { AnsiConsole.Markup(GradientMarkup.Text($"ValidateRegistrationCode Method at : {FN_ValidateRegistrationCode.Method} ... ")); FN_ValidateRegistrationCode.Instructions = new Instruction[] { Instruction.Create(OpCodes.Ldc_I4_0), Instruction.Create(OpCodes.Ret) }; p.Patch(FN_ValidateRegistrationCode); AnsiConsole.MarkupLine("[Green]Success[/]"); } targets = p.FindMethodsByArgumentSignatureExact(CLS_LicenseManager, "System.Boolean", "System.Boolean"); FN_IsRegisteredFromBackgroundThread = targets.FirstOrDefault(); if (FN_IsRegisteredFromBackgroundThread != null) { AnsiConsole.Markup(GradientMarkup.Text($"IsRegisteredFromBackgroundThread Method at : {FN_IsRegisteredFromBackgroundThread.Method} ... ")); p.WriteReturnBody(FN_IsRegisteredFromBackgroundThread, true); AnsiConsole.MarkupLine("[Green]Success[/]"); } AnsiConsole.Markup(GradientMarkup.Text("Saving patched file... ")); p.Save(true); AnsiConsole.MarkupLine("[Green]Success[/]"); AnsiConsole.MarkupLine($"[bold]Edition: [yellow]UltimatePlus[/][/]"); var email = AnsiConsole.Ask <string>("Enter [green]email address[/] to register"); AnsiConsole.MarkupLine($"[bold red on silver]Serial Number: {GenerateSerial("UltimatePlus", email)}[/]"); Console.ReadKey(); }