public void TestFixtureSetUp() { var client = DriverTestConfiguration.Client; var db = client.GetDatabase(DriverTestConfiguration.DatabaseNamespace.DatabaseName); db.DropCollectionAsync(DriverTestConfiguration.CollectionNamespace.CollectionName).GetAwaiter().GetResult(); _collection = db.GetCollection<ZipEntry>(DriverTestConfiguration.CollectionNamespace.CollectionName); // This is a subset of the data from the mongodb docs zip code aggregation examples _collection.InsertManyAsync(new[] { new ZipEntry { Zip = "01053", City = "LEEDS", State = "MA", Population = 1350 }, new ZipEntry { Zip = "01054", City = "LEVERETT", State = "MA", Population = 1748 }, new ZipEntry { Zip = "01056", City = "LUDLOW", State = "MA", Population = 18820 }, new ZipEntry { Zip = "01057", City = "MONSON", State = "MA", Population = 8194 }, new ZipEntry { Zip = "36779", City = "SPROTT", State = "AL", Population = 1191 }, new ZipEntry { Zip = "36782", City = "SWEET WATER", State = "AL", Population = 2444 }, new ZipEntry { Zip = "36783", City = "THOMASTON", State = "AL", Population = 1527 }, new ZipEntry { Zip = "36784", City = "THOMASVILLE", State = "AL", Population = 6229 }, }).GetAwaiter().GetResult(); }
public async void InsertDocumentsInCollection(List<BsonDocument> documents, IMongoCollection<BsonDocument> collection) { await collection.InsertManyAsync(documents); }
private static void AddMovies(IMongoCollection<Movie> collection) { var movies = new List<Movie> { new Movie { Title = "The Perfect Developer", Category = "SciFi", Minutes = 118 }, new Movie { Title = "Lost In Frankfurt am Main", Category = "Horror", Minutes = 122 }, new Movie { Title = "The Infinite Standup", Category = "Horror", Minutes = 341 } }; //collection.InsertBatch(movies); collection.InsertManyAsync(movies).Wait(); }
protected override void Execute(IMongoCollection<BsonDocument> collection, BsonDocument outcome, bool async) { if (async) { collection.InsertManyAsync(_documents).GetAwaiter().GetResult(); } else { collection.InsertMany(_documents); } }
public async Task BatchAddAsync(List <TEntity> l) { foreach (var t in l) { if (t is ICreateDate createdOn) { var created = createdOn.CreatedDate; if (created == default(DateTime)) { createdOn.CreatedDate = DateTime.Now; } } } var insertManyAsync = DbSet?.InsertManyAsync(l); if (insertManyAsync != null) { await insertManyAsync; } }
public Task InsertManyAsync(IEnumerable <T> documents, InsertManyOptions options = null, CancellationToken cancellationToken = default(CancellationToken)) { return(_Repository.InsertManyAsync(documents, options, cancellationToken)); }
/// <summary> /// Insert two documents (SQL=row) in the collection (SQL=table) as a single operation. /// </summary> private static async Task InsertManyAsync(IMongoCollection<BsonDocument> collection) { Console.WriteLine("\n++ Insert two documents (SQL=row) in the collection (SQL=table) as a single operation."); var document1 = new BsonDocument(newObj); // [MONGODB-DEMO] NoSQL is Unstructured (schemaless) - document1 and document2 have different set of key-value pairs. // [MONGODB-DEMO] There is no unique key '_id' (BsonObjectId) in document2 - will be generated by MongoDB automatically. var document2 = new BsonDocument { //{ "_id", ObjectId.GenerateNewId() }, { "Name", "Sergii"}, { "Surname", "Shevchenko"}, { "Extra info", "Phone: 3432 523 59"} }; await collection.InsertManyAsync(new [] {document1, document2}); }
public virtual async Task CreateAll(IEnumerable <TEntity> entities) { await TEntityCollection.InsertManyAsync(entities); }
/// <inheritdoc /> public Task AddAsync(IEnumerable <T> documents) { return(_mongoCollection.InsertManyAsync(documents)); }
public Task InsertRangeAsync(IEnumerable <TEntity> entities) { return(_collection.InsertManyAsync(entities)); }
private async Task CreateSamplePosts() { var postList = new List <Post>() { new Post() { Title = "First Post", Public = true, CoverImagePath = "", Excerpt = "Define the underlying", Content = "Define the underlying principles that drive decisions and strategy for your design language bleeding edge onward and upward" }, new Post() { Title = "Second Post", Public = true, CoverImagePath = "", Excerpt = "so what's our", Content = "so what's our go to market strategy?. Customer centric all hands on deck yet where the metal hits the meat define" }, new Post() { Title = "Not visible", Public = false, CoverImagePath = "", // blank Excerpt = "not important", Content = "not important, you should not see this post" }, new Post() { Title = "Third post", Public = true, CoverImagePath = "", // blank Excerpt = "the underlying", Content = "the underlying principles that drive decisions and strategy for your design language not the long pole", Deleted = true // this one should also not be visible }, new Post() { Title = "Fourth post", Public = true, CoverImagePath = "", // blank Excerpt = "in the future", Content = "Post scheduling made super easy", Created = DateTime.Now.AddMinutes(3) // Post scheduling made easy }, new Post() { Title = "Markdown Test", Public = true, CoverImagePath = "", // blank Excerpt = "Let's see what markdown can do", Content = "# Hello world\n" + "**Lorem** ipsum dolor sit" + "amet, *consectetur* adipiscing elit. Sed" + "eu est nec metus luctus tempus. Pellentesque" + "at elementum sapien, ac faucibus sem" + "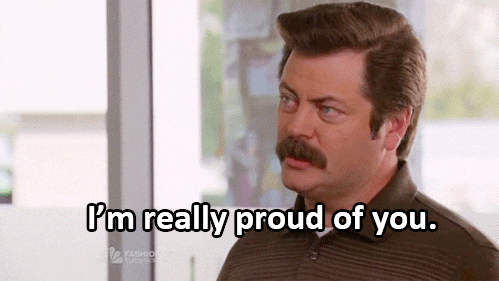" } }; if (await _posts.CountDocumentsAsync(p => true) == 0) { await _posts.InsertManyAsync(postList); } }
public async Task InsertAsync(List <TData> entitys) { await _collection.InsertManyAsync(entitys); }
public async Task CreateMovieAsync() { /* There are two methods for writing documents with the MongoDB * driver: InsertOne and InsertMany, as well as the Async versions * of those two methods. In this lesson, I'll show you how to use * the Async methods in a couple of different ways. * * Let's start with a simple example. We want to add a new movie * theater to the "theaters" collection. Let's first take a look at * the Theater mapping class. It has Id, TheaterId, and Location * properties. The Location property is an object that contains an * Address object and Geo information, which we won't worry about * in this lesson. * * With that bit of information, let's create a new instance of the * Theater class, using the constructor that takes in a TheaterId and * various Address components: * */ var newTheater = new Theater(27777, "4 Privet Drive", "Little Whinging", "LA", "343434"); // And now we call InsertOneAsync() to add it to the collection: await _theatersCollection.InsertOneAsync(newTheater); /* Here's something you should be aware of. In the constructor for * the Theater object, we don't set the Id property. Instead, * we let MongoDB create one and assign it for us as part of the * Insert call. We can check that property as soon as the InsertOne * returns: */ Assert.IsNotEmpty(newTheater.Id.ToString()); /* Adding multiple theaters to the collection is just as easy. * Let's add a group of 3 new theaters that have just been built. * First, we create the Theater objects: */ var theater1 = new Theater(27017, "1 Foo Street", "Dingledongle", "DE", "45678"); var theater2 = new Theater(27018, "234 Bar Ave.", "Wishywashy", "WY", "87654"); var theater3 = new Theater(27019, "75 Birthday Road", "Viejo Amigo", "CA", "99887"); /* And then we call InsertManyAsync, passing in a new List of * those Theater objects: */ await _theatersCollection.InsertManyAsync( new List <Theater>() { theater1, theater2, theater3 } ); /* Let's make sure everything worked. */ var newTheaters = await _theatersCollection.Find <Theater>( Builders <Theater> .Filter.Gte(t => t.TheaterId, 27017) & Builders <Theater> .Filter.Lte(t => t.TheaterId, 27019)) .ToListAsync(); Assert.AreEqual(3, newTheaters.Count); Assert.AreEqual("1 Foo Street", newTheaters.First().Location.Address.Street1); Assert.AreEqual("Viejo Amigo", newTheaters.Last().Location.Address.City); /* So that's all there is to writing new documents to a MongoDB * collection. In the next lesson, we'll look at updating * existing documents. */ }
public async static void InsertManyAsync(IMongoCollection<BsonDocument> collection, IEnumerable<BsonDocument> input) { await collection.InsertManyAsync(input); }
/// <inheritdoc/> public Task InsertManyAsync(IEnumerable <T> documents, InsertManyOptions options = null, CancellationToken cancellationToken = default) { return(_actualCollection.InsertManyAsync(documents, options, cancellationToken)); }
protected override async Task <int> InsertAsyncInternal(IEnumerable <TItem> items, CancellationToken token = default) { await _collection.InsertManyAsync(items, new InsertManyOptions(), token); return(items.Count()); }
public Task InsertStoriesAsync(IEnumerable <StoryModel> stories, CancellationToken cancellationToken) { _ = stories ?? throw new ArgumentNullException(nameof(stories)); return(_storyCollection.InsertManyAsync(stories, cancellationToken: cancellationToken)); }
protected override Task WriteMessagesAsync(IEnumerable <IPersistentRepresentation> messages) { var entries = messages.Select(ToJournalEntry).ToList(); return(_collection.InsertManyAsync(entries)); }
public async Task SaveAsync(IEnumerable <TvMazeScrapperDp.Core.Models.Show> shows, CancellationToken cancellationToken) { await _showCollection.InsertManyAsync(shows.Select(_mapper.Map <Show>), new InsertManyOptions(), cancellationToken); }
public async Task AddAsync(IEnumerable <T> tlist) { await _collection.InsertManyAsync(tlist); }
/// <summary> /// Inserts multiple documents at once into this collection (see also Insert to insert a single document). /// </summary> /// <typeparam name="T">The default document type for this collection.</typeparam> /// <param name="collection">An instance of MongoCollection.</param> /// <param name="documents">The documents to insert.</param> /// <returns>A list of WriteConcernResults (or null if WriteConcern is disabled).</returns> public virtual void InsertBatch <T>(IMongoCollection <T> collection, IEnumerable <T> documents) { lock (_lockObject) collection.InsertManyAsync(documents); }
public async Task InsertAsync(IEnumerable <T> documents) { await collection.InsertManyAsync(documents); }
public void FillColection(List <Track> tracks) { collection.InsertManyAsync(tracks); }
public async Task CreateAsync(List <T> entities) => await _collection.InsertManyAsync(entities);
static async void UserDummyData(IMongoCollection <User> user) { var newUsers = new List <User> { new User { Id = "100000000000000000000001", Name = "Mikkel", Gender = "Male", Age = 31, BlockList = new List <string>() { "100000000000000000000003" }, Followers = new List <string> { "100000000000000000000002" }, Following = new List <string> { "100000000000000000000002" }, Circles = new List <string> { "400000000000000000000001" }, }, new User { Id = "100000000000000000000002", Name = "Frodi", Gender = "Male", Age = 32, BlockList = new List <string>() { "100000000000000000000003" }, Followers = new List <string> { "100000000000000000000001" }, Following = new List <string> { "100000000000000000000001" }, Circles = new List <string> { "400000000000000000000001" }, }, new User { Id = "100000000000000000000003", Name = "Outsider", Gender = "Male", Age = 30, BlockList = new List <string>(), Followers = new List <string> { }, Following = new List <string> { "100000000000000000000001", "100000000000000000000002" }, Circles = new List <string> { }, }, new User { Id = "100000000000000000000004", Name = "Nanna", Gender = "Feale", Age = 25, BlockList = new List <string>(), Followers = new List <string> { }, Following = new List <string> { }, Circles = new List <string> { "400000000000000000000001" }, } }; await user.InsertManyAsync(newUsers); }
public async Task InsertAsync(IEnumerable <TEntity> entities) { await _mongoCollection.InsertManyAsync(entities); }
/// <summary> /// Async Insert entities /// </summary> /// <param name="entities">Entities</param> public virtual async Task <IEnumerable <T> > InsertAsync(IEnumerable <T> entities) { await _collection.InsertManyAsync(entities); return(entities); }
protected override Task ExecuteAsync(IMongoCollection<BsonDocument> collection, BsonDocument outcome) { return collection.InsertManyAsync(_documents); }
public virtual async Task InsertManyAsync(ICollection <TDocument> documents) { await _collection.InsertManyAsync(documents); }
public async Task AddManyAsync(List <TEntity> items) { await Collection.InsertManyAsync(items); }
public Task CreateDocumentAsync(IEnumerable <T> documents) { return(_collection.InsertManyAsync(documents)); }
static async Task AddDocuments(IMongoCollection<Person> collection) { var testdoc = new Person ("jones"); testdoc.Age = 30; testdoc.Profession = "hacker"; var nestedArray = new List<string>(); nestedArray.Add("color"); nestedArray.Add("red"); testdoc.Preferences = nestedArray; await collection.InsertOneAsync(testdoc); Console.WriteLine("Adding:" + testdoc.ToBsonDocument().ToString()); var testdoc2 = new Person("jones"); testdoc2.Age = 50; testdoc2.Profession = "retired hacker"; await collection.InsertOneAsync(testdoc2); Console.WriteLine("Adding:" + testdoc2.ToBsonDocument().ToString()); var doc2 = new Person("Smith"); var doc3 = new Person("White"); await collection.InsertManyAsync(new[] { doc2, doc3 }); Console.WriteLine("Adding:" + doc2.ToBsonDocument().ToString() + "\nAdding:" + doc3.ToBsonDocument().ToString()); }
public async Task AddRangeAsync(IEnumerable <T> items) { await _collection.InsertManyAsync(items); }
public async Task <List <Products> > CreateRange(List <Products> obj) { await _products.InsertManyAsync(obj); return(obj); }
private async Task InitializeCollectionAsync(IMongoCollection<BsonDocument> collection, List<BsonDocument> documents) { await collection.DeleteManyAsync(new BsonDocument()); if (documents.Count > 0) { await collection.InsertManyAsync(documents); } }
public static async Task InsertMessages(IEnumerable <MessageRecord> msgs) { await _messagesCollection.InsertManyAsync(msgs); }
public static async Task InsertPastas(IEnumerable <PastaRecord> pastas) { await _pastasCollection.InsertManyAsync(pastas); }
public static async Task AddRangeAsync <T>(this IMongoCollection <T> collection, IEnumerable <T> values, CancellationToken cancellationToken = default) { await collection.InsertManyAsync(values, cancellationToken : cancellationToken); }
public void Setup() { _runner = MongoDbRunner.Start(); var mongoUrlBuilder = new MongoUrlBuilder(_runner.ConnectionString); mongoUrlBuilder.DatabaseName = "AutomatedTest"; //setup mongo database var client = new MongoClient(mongoUrlBuilder.ToMongoUrl()); Database = client.GetDatabase(mongoUrlBuilder.DatabaseName); Collection = Database.GetCollection<RootEntity>("Root"); ContainsNotProvidedTagsEntityId = ObjectId.GenerateNewId(); SubEntityId = ObjectId.GenerateNewId(); //add mock data var entities = new List<RootEntity>() { new RootEntity() { Id = ObjectId.GenerateNewId(), Tags = new List<string>() {"tag1"}, SubCollection = new List<SubEntity>() { new SubEntity() { Id = SubEntityId } } }, new RootEntity() { Id = ContainsNotProvidedTagsEntityId, Tags = new List<string>() {"tag1", "tag2"}, SubCollection = new List<SubEntity>() { new SubEntity() { Id = SubEntityId } } } }; Collection.InsertManyAsync(entities).Wait(); }
public virtual Task InsertMany(IEnumerable <T> entities, CancellationToken token = default) { return(_collection.InsertManyAsync(entities, cancellationToken: token)); }