public void QueryProcessor_TestExecuteQuery() { //Uses Moq to test the Query Processor logic //Arrange: var connectionInfo = CreateConnectionInfo(); var query = new Query(); query.RootEntity.ObjectDefinitionFullName = "Registrant"; //use this to return a pre-determined set of data that we control: var registrant = CreateRegistrants(); var webinar = CreateUpcomingWebinars(); //set up the fake data: var clientMoq = new Mock<IGoToWebinarClient>(); clientMoq.Setup(client => client.GetUpcomingWebinars(connectionInfo["AccessToken"], connectionInfo["OrganizerKey"])).Returns(webinar); clientMoq.Setup(client => client.GetRegistrants(connectionInfo["AccessToken"], connectionInfo["OrganizerKey"], "1235813")).Returns(registrant); //create our the instance of the queryprocessor using our Moq class: var queryProcessor = new QueryProcessor(connectionInfo, clientMoq.Object); //Act: IEnumerable<DataEntity> dataEntities = queryProcessor.ExecuteQuery(query); //Assert: //since we're returning a predeternimed list of registrants, //we're only testing the translation of registrants to dataentities. Assert.AreEqual(2, dataEntities.Count()); Assert.IsTrue(dataEntities.Any(entity => entity.Properties.Any(field => field.Value == "jim"))); }
public ModulesSource(WikiInfo wiki, string propsDefaultsPath = null) { Dictionary<string, XElement> propsDefaults = null; if (propsDefaultsPath != null) propsDefaults = XDocument.Load(propsDefaultsPath).Root.Elements().ToDictionary(e => (string)e.Attribute("name")); m_processor = new QueryProcessor<ParamInfo>( wiki, new QueryTypeProperties<ParamInfo>( "paraminfo", "", null, null, new TupleList<string, string> { { "action", "paraminfo" } }, null, e => ParamInfo.Parse(e, propsDefaults))); }
public void QueryProcessor_TestExecuteQuery_NullQueryName() { //Test a 'bad' condition to make sure the code doesn't break: //Arrange: var connectionInfo = CreateConnectionInfo(); var query = new Query(); query.RootEntity.ObjectDefinitionFullName = null; var clientMoq = new Mock<IGoToWebinarClient>(); var queryProcessor = new QueryProcessor(connectionInfo, clientMoq.Object); //Act: IEnumerable<DataEntity> dataEntities = queryProcessor.ExecuteQuery(query); //Assert: Assert.IsNotNull(dataEntities); }
private static void Main(string[] args) { SemanticTypeActions<QueryToken> queryActions = new SemanticTypeActions<QueryToken>(CompiledGrammar.Load(typeof(QueryToken), "Query.cgt")); queryActions.Initialize(true); SemanticTypeActions<ExpressionToken> expressionActions = new SemanticTypeActions<ExpressionToken>(CompiledGrammar.Load(typeof(ExpressionToken), "Expression.cgt")); expressionActions.Initialize(true); string input = "VIEW a WHERE x = 20 ORDER BY y"; using (StringReader reader = new StringReader(input)) { QueryProcessor processor = new QueryProcessor(new QueryTokenizer(reader, queryActions, expressionActions)); ParseMessage message = processor.ParseAll(); Console.WriteLine("Parsing result: "+message); if (message != ParseMessage.Accept) { Console.WriteLine(input); Console.Write(new string(' ', (int)((IToken)processor.CurrentToken).Position.Index)); Console.WriteLine('^'); } } Console.ReadKey(false); }
public LoginResult Login(string name, string password, string token = null) { var queryProcessor = new QueryProcessor<LoginResult>( m_info, new QueryTypeProperties<LoginResult>( "login", "lg", null, null, new TupleList<string, string> { { "action", "login" } }, null, LoginResult.Parse)); var parameters = QueryParameters.Create<LoginResult>(); if (name != null) parameters = parameters.AddSingleValue("name", name); if (password != null) parameters = parameters.AddSingleValue("password", password); if (token != null) parameters = parameters.AddSingleValue("token", token); return queryProcessor.ExecuteSingle(parameters); }
public void QueryProcessor_TestExecute_DateFilteredWebinar() { //We're not testing to see if GoToWebinar filters correctly, //but that our Query Builder does the right stuff //Arrange var connectionInfo = CreateConnectionInfo(); var query = CreateDateFilterQuery(); var webinars = CreateWebinars(); var clientMoq = new Mock<IGoToWebinarClient>(); clientMoq.Setup(client => client.GetWebinars(connectionInfo["AccessToken"], connectionInfo["OrganizerKey"], new DateTime(2010, 01, 01), new DateTime(2013, 01, 01))).Returns(webinars); var queryProcessor = new QueryProcessor(connectionInfo, clientMoq.Object); //Act: IEnumerable<DataEntity> entities = queryProcessor.ExecuteQuery(query); //Assert Assert.AreEqual(1, entities.Count()); }
public ActionResult RecordLink(string pid, string rid, string familyId, string compareFamilyId) { DefaultComparisonEngineConfigurationFileLocation = Path.Combine(Request.PhysicalApplicationPath, DefaultComparisonEngineConfigurationFileLocation); NetworkClient client = new NetworkClient(new DataProperties()); personRankConnectionPool = client.CreatePool(new ServerId[] { new ServerId("10.8.129.1:80") }, 60000, 60000); queryProcessor = new QueryProcessor(personRankConnectionPool); // TODO: Send person id int censusYear = CensusHelper.GetCensusYear(int.Parse(rid)); int databaseId = CensusHelper.GetDatabaseId(censusYear, "prev"); int searchCensusYear = CensusHelper.GetCensusYear(databaseId); QueryResultList results; try { results = queryProcessor.ExecuteQuery(string.Format("{0}:{1}", pid, rid)); } catch { return new JsonResult { Data = string.Empty, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; } Family family = results.PersonContainer.GetFamilies().Where(x => x.Id == familyId).FirstOrDefault(); Family compareFamily = results.PersonContainer.GetFamilies().Where(x => x.Id == compareFamilyId).FirstOrDefault(); // TODO: loop through the family IList<PersonModel.Person> personList = new List<PersonModel.Person>(); IList<PersonModel.Person> personList2 = new List<PersonModel.Person>(); foreach (RelationshipPointer child in family.Children.ChildPointers) { PersonModel.Person person = results.PersonContainer.GetPerson(child.Id); foreach (RelationshipPointer compareChild in compareFamily.Children.ChildPointers) { PersonModel.Person comparePerson = results.PersonContainer.GetPerson(child.Id); FeatureComparisonEngine comparisonEngine = new FeatureComparisonEngine(DefaultComparisonEngineConfigurationFileLocation); ComparisonResult result = comparisonEngine.ComparePeople(results.PersonContainer, person, comparePerson); } } return new JsonResult { Data = string.Empty, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; }
public List <ModelResult> Parse(string query) { // Preprocess the query query = QueryProcessor.Preprocess(query, caseSensitive: true); var extractionResults = new List <ModelResult>(); try { foreach (var p in ExtractorParserDic) { var extractor = p.Key; var parser = p.Value; var extractedResults = extractor.Extract(query); var parsedResults = new List <ParseResult>(); foreach (var result in extractedResults) { var parseResult = parser.Parse(result); if (parseResult.Value is List <ParseResult> ) { parsedResults.AddRange((List <ParseResult>)parseResult.Value); } else { parsedResults.Add(parseResult); } } var modelResults = parsedResults.Select(o => new ModelResult { Start = o.Start.Value, End = o.Start.Value + o.Length.Value - 1, Resolution = (o.Value is CurrencyUnitValue) ? new SortedDictionary <string, object> { { ResolutionKey.Value, ((CurrencyUnitValue)o.Value).Number }, { ResolutionKey.Unit, ((CurrencyUnitValue)o.Value).Unit }, { ResolutionKey.IsoCurrency, ((CurrencyUnitValue)o.Value).IsoCurrency }, } : (o.Value is UnitValue) ? new SortedDictionary <string, object> { { ResolutionKey.Value, ((UnitValue)o.Value).Number }, { ResolutionKey.Unit, ((UnitValue)o.Value).Unit }, } : new SortedDictionary <string, object> { { ResolutionKey.Value, (string)o.Value }, }, Text = o.Text, TypeName = ModelTypeName, }).ToList(); foreach (var result in modelResults) { bool shouldAdd = true; foreach (var extractionResult in extractionResults) { if (extractionResult.Start == result.Start && extractionResult.End == result.End) { shouldAdd = false; } } if (shouldAdd) { extractionResults.Add(result); } } } } catch (Exception) { // Nothing to do. Exceptions in parse should not break users of recognizers. // No result. } return(extractionResults); }
public int GetMeasurementsWithoutDiff() { return(QueryProcessor.Execute(new GetMeasurementsWithoutDiffQuery())); }
protected EntityManagerBase(DbContextFactory dbContextFactory, QueryProcessor queryProcessor) { _dbContextFactory = dbContextFactory; QueryProcessor = queryProcessor; }
public async Task when_processing_the_query() { FakeQueryTypeCollection = new Mock <IQueryTypeCollection>(); FakeServiceProvider = new Mock <IServiceProvider>(); Subject = new QueryProcessor(FakeQueryTypeCollection.Object, FakeServiceProvider.Object); async Task should_invoke_the_correct_query_handler() { var fakeQueryHandler = new FakeQueryHandler(x => { Expected = x; return(new FakeResult()); }); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler); var query = new FakeQuery(); await Subject.ProcessAsync(query); Expected.Should().Be(query); } async Task should_return_the_result_from_the_query_handler() { var expected = new FakeResult(); var query = new FakeQuery(); var fakeQueryHandler = new FakeQueryHandler(x => expected); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler); var result = await Subject.ProcessAsync(query); result.Should().Be(expected); } void should_throw_exception_if_the_query_handler_is_not_found() { var query = new Mock <IQuery <FakeResult> >().Object; Subject.Awaiting(async x => await x.ProcessAsync(query)).Should() .Throw <QueryProcessorException>() .WithMessage($"The query handler for '{query}' could not be found"); } void should_throw_exception_if_the_query_type_is_not_found() { var queryName = "NotFoundQuery"; var json = JObject.Parse("{}"); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, json)).Should() .Throw <QueryProcessorException>() .WithMessage("The query type 'NotFoundQuery' could not be found"); } void should_throw_exception_if_the_json_is_invalid() { var queryName = "FakeQuery"; FakeQueryTypeCollection.Setup(x => x.GetType(queryName)).Returns(typeof(FakeQuery)); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, (JObject)null)).Should() .Throw <QueryProcessorException>() .WithMessage("The json could not be converted to an object"); } void should_throw_exception_if_the_dictionary_is_invalid() { var queryName = "FakeQuery"; FakeQueryTypeCollection.Setup(x => x.GetType(queryName)).Returns(typeof(FakeQuery)); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, (IDictionary <string, string>)null)).Should() .Throw <QueryProcessorException>() .WithMessage("The dictionary could not be converted to an object"); } }
public Task <IResult <List <IpWarmup> > > Execute() { return(QueryProcessor.Process <IpWarmup, IIpWarmupListQuery, IpWarmupListQuery>(this, context => context.IpWarmups())); }
public Task <IResult <List <WebhookParseSettings> > > Execute() { return(QueryProcessor .Process <WebhookParseSettings, IWebhookParseSettingsListQuery, WebhookParseSettingsListQuery>(this, context => context.WebhookParseSettings())); }
public Task <IResult <MailFooterSetting> > Execute() { return(QueryProcessor .Process <MailFooterSetting, IMailFooterSettingSingleQuery, MailFooterSettingSingleQuery>(this, context => context.MailFooterSetting())); }
public Task <IResult <EmailReport> > Execute() { return(QueryProcessor .Process <EmailReport, IInvalidEmailAddressSingleQuery, InvalidEmailAddressSingleQuery>(this, context => context.InvalidEmailAddressByEmailAddress(EmailAddress))); }
public FriendRequestManager(DbContextFactory dbContextFactory, QueryProcessor queryProcessor) : base(dbContextFactory, queryProcessor) { }
public Task <IResult <BrandedLink> > Execute() { return(QueryProcessor.Process <BrandedLink, IBrandedLinkSingleQuery, BrandedLinkSingleQuery>(this, context => context.BrandedLinkById(Id))); }
public async Task when_processing_the_query() { FakeQueryTypeCollection = new Mock <IQueryTypeCollection>(); FakeServiceProvider = new Mock <IServiceProvider>(); Subject = new QueryProcessor(FakeQueryTypeCollection.Object, FakeServiceProvider.Object); async Task should_invoke_the_correct_query_handler() { FakeQuery expectedQuery = null; var fakeQueryHandler = new FakeQueryHandler(x => { expectedQuery = x; return(new FakeResult()); }); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler); var query = new FakeQuery(); await Subject.ProcessAsync(query); query.Should().Be(expectedQuery); } async Task should_create_the_query_from_a_string() { var expectedQueryType = typeof(FakeQuery); var fakeQueryHandler = new Mock <IQueryHandler <FakeQuery, FakeResult> >(); FakeQueryTypeCollection.Setup(x => x.GetType(expectedQueryType.Name)).Returns(expectedQueryType); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler.Object); await Subject.ProcessAsync <FakeResult>(expectedQueryType.Name, "{}"); fakeQueryHandler.Verify(x => x.HandleAsync(It.IsAny <FakeQuery>())); } async Task should_create_the_query_from_a_dictionary() { var expectedQueryType = typeof(FakeQuery); var fakeQueryHandler = new Mock <IQueryHandler <FakeQuery, FakeResult> >(); FakeQueryTypeCollection.Setup(x => x.GetType(expectedQueryType.Name)).Returns(expectedQueryType); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler.Object); await Subject.ProcessAsync <FakeResult>(expectedQueryType.Name, new Dictionary <string, IEnumerable <string> >()); fakeQueryHandler.Verify(x => x.HandleAsync(It.IsAny <FakeQuery>())); } async Task should_create_a_complex_query_from_a_dictionary() { var expectedQueryType = typeof(FakeComplexQuery); FakeComplexQuery expectedQuery = null; var fakeQueryHandler = new FakeComplexQueryHandler(x => { expectedQuery = x; return(new List <FakeResult>()); }); FakeQueryTypeCollection.Setup(x => x.GetType(expectedQueryType.Name)).Returns(expectedQueryType); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeComplexQuery, IEnumerable <FakeResult> >))).Returns(fakeQueryHandler); var query = new Dictionary <string, IEnumerable <string> > { { "String", new[] { "Value" } }, { "Int", new[] { "1" } }, { "Bool", new[] { "true" } }, { "DateTime", new[] { "2018-07-06" } }, { "Guid", new[] { "3B10C34C-D423-4EC3-8811-DA2E0606E241" } }, { "NullableDouble", new[] { "2.1" } }, { "UndefinedProperty", new[] { "should_not_be_used" } }, { "Array", new[] { "1", "2" } }, { "IEnumerable", new[] { "3", "4" } }, { "List", new[] { "5", "6" } } }; await Subject.ProcessAsync <IEnumerable <FakeResult> >(expectedQueryType.Name, query); expectedQuery.String.Should().Be("Value"); expectedQuery.Int.Should().Be(1); expectedQuery.Bool.Should().Be(true); expectedQuery.DateTime.Should().Be(DateTime.Parse("2018-07-06")); expectedQuery.Guid.Should().Be(new Guid("3B10C34C-D423-4EC3-8811-DA2E0606E241")); expectedQuery.NullableDouble.Should().Be(2.1); expectedQuery.Array.Should().Equal(1, 2); expectedQuery.IEnumerable.Should().Equal(3, 4); expectedQuery.List.Should().Equal(5, 6); } async Task should_return_the_result_from_the_query_handler() { var expected = new FakeResult(); var fakeQueryHandler = new FakeQueryHandler(x => expected); FakeServiceProvider.Setup(x => x.GetService(typeof(IQueryHandler <FakeQuery, FakeResult>))).Returns(fakeQueryHandler); var query = new FakeQuery(); var result = await Subject.ProcessAsync(query); result.Should().Be(expected); } void should_throw_exception_if_the_query_handler_is_not_found() { var query = new Mock <IQuery <FakeResult> >().Object; Subject.Awaiting(async x => await x.ProcessAsync(query)).Should() .Throw <QueryProcessorException>() .WithMessage($"The query handler for '{query}' could not be found"); } void should_throw_exception_if_the_query_type_is_not_found() { var queryName = "NotFoundQuery"; var json = JObject.Parse("{}"); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, json)).Should() .Throw <QueryProcessorException>() .WithMessage("The query type 'NotFoundQuery' could not be found"); } void should_throw_exception_if_the_json_is_invalid() { var queryName = "FakeQuery"; FakeQueryTypeCollection.Setup(x => x.GetType(queryName)).Returns(typeof(FakeQuery)); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, (JObject)null)).Should() .Throw <QueryProcessorException>() .WithMessage("The json could not be converted to an object"); } void should_throw_exception_if_the_dictionary_is_invalid() { var queryName = "FakeQuery"; FakeQueryTypeCollection.Setup(x => x.GetType(queryName)).Returns(typeof(FakeQuery)); Subject.Awaiting(async x => await x.ProcessAsync <object>(queryName, (IDictionary <string, IEnumerable <string> >)null)).Should() .Throw <QueryProcessorException>() .WithMessage("The dictionary could not be converted to an object"); } }
public List <ModelResult> Parse(string query) { var parsedNumbers = new List <ParseResult>(); // Preprocess the query query = QueryProcessor.Preprocess(query, caseSensitive: true); try { var extractResults = Extractor.Extract(query); foreach (var result in extractResults) { var parseResult = Parser.Parse(result); if (parseResult.Data is List <ParseResult> parseResults) { parsedNumbers.AddRange(parseResults); } else { parsedNumbers.Add(parseResult); } } } catch (Exception) { // Nothing to do. Exceptions in parse should not break users of recognizers. // No result. } return(parsedNumbers.Select(o => { var end = o.Start.Value + o.Length.Value - 1; var resolution = new SortedDictionary <string, object>(); if (o.Value != null) { resolution.Add(ResolutionKey.Value, o.ResolutionStr); } var extractorSupportsSubtype = ExtractorsSupportingSubtype.Exists(e => Extractor.GetType().ToString().Contains(e)); // Check if current extractor supports the Subtype field in the resolution // As some languages like German, we miss handling some subtypes between "decimal" and "integer" if (!string.IsNullOrEmpty(o.Type) && Constants.ValidSubTypes.Contains(o.Type) && extractorSupportsSubtype) { resolution.Add(ResolutionKey.SubType, o.Type); } var type = string.Empty; // for ordinal and ordinal.relative // Only support "ordinal.relative" for English for now if (ModelTypeName.Equals(Constants.MODEL_ORDINAL)) { if (o.Metadata != null && o.Metadata.IsOrdinalRelative) { type = $"{ModelTypeName}.{Constants.RELATIVE}"; } else { type = ModelTypeName; } resolution.Add(ResolutionKey.Offset, o.Metadata.Offset); resolution.Add(ResolutionKey.RelativeTo, o.Metadata.RelativeTo); } else { type = ModelTypeName; } return new ModelResult { Start = o.Start.Value, End = end, Resolution = resolution, Text = o.Text, TypeName = type, }; }).ToList()); }
public QueryProcessorTests() { _handler = new Mock <IQueryHandler <FakeQuery, object> >(); _resolver = new Mock <IResolver>(); _processor = new QueryProcessor(_resolver.Object); }
public T Handle([NotNull] CommonRuleChildElementQuery <T> query) { var commonRule = QueryProcessor.Process(new CommonRuleQuery(query.TypiconId, query.Name)); return(commonRule?.GetRule <T>(query.RuleSerializer)); }
public Task <IResult <List <SpamReportedEmailAddress> > > Execute() { return(QueryProcessor .Process <SpamReportedEmailAddress, ISpamReportedEmailAddressListQuery, SpamReportedEmailAddressListQuery >(this, context => context.SpamReportedEmailAddresses(this))); }
public Task <IResult <List <RemainingIpAddress> > > Execute() { return(QueryProcessor .Process <RemainingIpAddress, IRemainingIpAddressListQuery, RemainingIpAddressListQuery>(this, context => context.RemainingIpAddresses())); }
public Task <IResult <UserAccount> > Execute() { return(QueryProcessor.Process <UserAccount, IUserAccountSingleQuery, UserAccountSingleQuery>(this, context => context.UserAccount())); }
public GetMeasurementAverageValueResponse GetAverageValues() { return(QueryProcessor.Execute(new GetMeasurementAverageValueQuery())); }
public Task <IResult <List <MailSetting> > > Execute() { return(QueryProcessor.Process <MailSetting, IMailSettingListQuery, MailSettingListQuery>(this, context => context.MailSettings(this))); }
public IEnumerable <GetMeterMeasurementTypesResponse> GetMeasurementTypes() { return(QueryProcessor.Execute(new GetMeterMeasurementTypesQuery())); }
public Task <IResult <CampaignSchedule> > Execute() { return(QueryProcessor.Process <CampaignSchedule, ICampaignScheduleSingleQuery, CampaignScheduleSingleQuery>( this, context => context.CampaignScheduleByCampaignId(CampaignId))); }
public async Task <IResult <List <SubUser> > > Execute() { var subUserListResult = await QueryProcessor.Process <SubUser, ISubUserListQuery, SubUserListQuery>(this, context => context.SubUsers(this)); if (subUserListResult.IsSuccess && subUserListResult.Data.Any()) { foreach (var subUser in subUserListResult.Data) { if (ReputationListQuery != null) { ReputationListQuery .UseContextOption(ContextOptionAction) .AddSubUser(subUser.UserName); var reputationResult = await ReputationListQuery.Execute(); if (reputationResult.IsSuccess) { subUser.Reputations = reputationResult.Data; } } if (MonitorSettingSingleQuery != null) { MonitorSettingSingleQuery .BySubUserName(subUser.UserName) .UseContextOption(ContextOptionAction); var monitorSettingResult = await MonitorSettingSingleQuery.Execute(); if (monitorSettingResult.IsSuccess) { subUser.MonitorSetting = monitorSettingResult.Data; } } Action <IContextOption> contextOption = option => option .OnBehalfOfSubUser(subUser.UserName) .UseApiKey(ContextOption?.ApiKey ?? _defaultApiKey); if (BlockedEmailAddressListQuery != null) { BlockedEmailAddressListQuery.UseContextOption(contextOption); var blockedEmailAddressListResult = await BlockedEmailAddressListQuery.Execute(); if (blockedEmailAddressListResult.IsSuccess) { subUser.BlockedEmailReports = blockedEmailAddressListResult.Data; } } if (BouncedEmailAddressListQuery != null) { BouncedEmailAddressListQuery.UseContextOption(contextOption); var blockedEmailAddressListResult = await BouncedEmailAddressListQuery.Execute(); if (blockedEmailAddressListResult.IsSuccess) { subUser.BlockedEmailReports = blockedEmailAddressListResult.Data; } } if (InvalidEmailAddressListQuery != null) { InvalidEmailAddressListQuery.UseContextOption(contextOption); var invalidEmailAddressListQuery = await InvalidEmailAddressListQuery.Execute(); if (invalidEmailAddressListQuery.IsSuccess) { subUser.InvalidEmailReports = invalidEmailAddressListQuery.Data; } } if (SpamReportedEmailAddressListQuery != null) { SpamReportedEmailAddressListQuery.UseContextOption(contextOption); var spamReportedEmailAddressListQuery = await SpamReportedEmailAddressListQuery.Execute(); if (spamReportedEmailAddressListQuery.IsSuccess) { subUser.SpamReportedEmails = spamReportedEmailAddressListQuery.Data; } } if (AlertListQuery != null) { AlertListQuery.UseContextOption(contextOption); var alertListQuery = await AlertListQuery.Execute(); if (alertListQuery.IsSuccess) { subUser.Alerts = alertListQuery.Data; } } if (ApiKeyListQuery != null) { ApiKeyListQuery.UseContextOption(contextOption); var apiKeyListQuery = await ApiKeyListQuery.Execute(); if (apiKeyListQuery.IsSuccess) { subUser.ApiKeys = apiKeyListQuery.Data; } } } } return(subUserListResult); }
/// <summary> /// Handles the custom message. The message will have the query information /// </summary> /// <param name="activity">The activity.</param> /// <returns></returns> private static async Task <string> HandleCustomMessage(Activity activity) { //Get natural language processed by LUIS var luisResponse = await LUISMetalQueryClient.ParseUserInput(activity.Text); var responseMessage = string.Empty; switch (luisResponse.intents[0].intent) { case "Greeting": responseMessage = "Hi there, how can I help you?"; return(responseMessage); case "Metal Sales": var showHelp = luisResponse.entities.Any(x => x.type == "help" || x.type == "greeting"); // should check intents not entities if (showHelp) { responseMessage = $"Hi I am **Metal Bot**, I can assist you with sales of Metal. {Environment.NewLine}You can ask me something like 'What is the average sale for 2010?' or 'What was the highest sale in USA,Widwest'. {Environment.NewLine}**Give me a try!** {Environment.NewLine}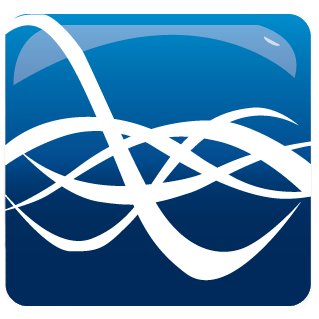"; return(responseMessage); } // what is the lowest for gold in 20/10/2017 var metals = luisResponse.entities.Where(x => x.type == "metal").Select(x => x.entity).ToList(); var numbers = luisResponse.entities.Where(x => x.type == "builtin.number").Select(x => x.resolution.value).ToList(); var dates = luisResponse.entities.Where(x => x.type == "builtin.datetimeV2.date").Select(x => x.resolution.date).ToList(); var parsedDates = dates.Select(x => x.Contains('-') ? DateTime.Parse(x) : new DateTime(int.Parse(x), 1, 1)).ToList(); var isPriceOriented = luisResponse.entities.Any(x => x.type == "cost"); var requestsLowest = luisResponse.entities.Any(x => x.type == "lowest"); var requestsHighest = luisResponse.entities.Any(x => x.type == "highest"); DateTime maxDate; DateTime minDate; using (var dataContext = new botEntities()) { maxDate = dataContext.prices.Max(x => x.date); minDate = dataContext.prices.Min(x => x.date); } if (parsedDates.Any() && parsedDates.All(x => !(minDate <= x && maxDate >= x))) { responseMessage = $"Sorry I only have data from {minDate:MMMM yyyy} to {maxDate:MMMM yyyy}"; return(responseMessage); } PriceFilterType priceFilter; if (requestsLowest) //Intentionally done first, in case both exist i.e worst expensive { priceFilter = PriceFilterType.Least; } else if (requestsHighest) { priceFilter = PriceFilterType.Most; } else { priceFilter = PriceFilterType.None; } //Question 'What is the cost of gold in January 2015' has 2015 as part of date and number numbers.RemoveAll(x => x.Length == 4 && dates.Any(d => d.Contains(x))); var hasMonths = false; if (dates.Any()) { hasMonths = dates.Any(x => x.Contains('-')); } var number = 1; //TODO: What if user gives more than one number if (numbers.Any()) { number = int.Parse(numbers.First()); } var queryDescriptor = new QueryDescriptor( isPriceOriented, metals, parsedDates, hasMonths ? TimelineConstraintLimit.Month : TimelineConstraintLimit.Year, priceFilter, !dates.Any(), number ); var result = QueryProcessor.Process(queryDescriptor); var failedToEvaluate = EvaluateResultStatus(out responseMessage, result.QueryResultType); if (failedToEvaluate) { return(responseMessage); } responseMessage = FormatResult(result.Prices.ToList(), number); break; case "Price": //what is the price of copper in April 2017? break; case "Average Price": //what is average price of gold in 2017? break; case "Help": responseMessage = "I can help you with finding the price of a metal in a given month, the average price over a year, the highest price and the lowest price."; break; case "None": responseMessage = "Sorry, I don't understand, perhaps try something like \"What was the price of gold in April 2017?\""; break; } return(responseMessage); }
public ActionResult FamilySearch(string pid, string rid, string dir) { NetworkClient client = new NetworkClient(new DataProperties()); personRankConnectionPool = client.CreatePool(new ServerId[] { new ServerId("10.8.129.1:80") }, 60000, 60000); queryProcessor = new QueryProcessor(personRankConnectionPool); // Get the census year from database id int censusYear = CensusHelper.GetCensusYear(int.Parse(rid)); // Get the previous/next census year int databaseId = CensusHelper.GetDatabaseId(censusYear, dir); if (databaseId == 0) { return new JsonResult { Data = string.Empty, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; } // Get the previous database id int searchCensusYear = CensusHelper.GetCensusYear(databaseId); QueryResultList results; try { results = queryProcessor.ExecuteQuery(string.Format("{0}:{1}", pid, rid)); } catch { return new JsonResult { Data = string.Empty, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; } List<SimpleFamily> familyResults = new List<SimpleFamily>(); List<Family> filteredResults = null; //#region new approach //foreach (QueryResult result in results) //{ // if (result.Person.Id.DatabaseId == databaseId) // { // var familyList = results.PersonContainer.GetFamilies(result.Person.Id); // if (familyList.Count > 1) // { // foreach (Family family in familyList) // { // SimpleFamily simpleFamily = new SimpleFamily(); // simpleFamily.Id = family.Id; // simpleFamily.CensusYear = searchCensusYear; // simpleFamily.Mother = SimplePerson.CreatePerson(results.PersonContainer.GetPerson(family.MotherId), simpleFamily.CensusYear); // simpleFamily.Father = SimplePerson.CreatePerson(results.PersonContainer.GetPerson(family.FatherId), simpleFamily.CensusYear); // foreach (RelationshipPointer child in family.Children.ChildPointers) // { // simpleFamily.Children.Add(SimplePerson.CreatePerson(results.PersonContainer.GetPerson(child.Id), simpleFamily.CensusYear)); // } // simpleFamily.FindPerson(results); // familyResults.Add(simpleFamily); // } // } // else // { // SimpleFamily simpleFamily = new SimpleFamily(); // if (result.Person.Gender == GenderType.Female) // { // simpleFamily.Mother = SimplePerson.CreatePerson(result.Person, censusYear); // } // else // { // simpleFamily.Father = SimplePerson.CreatePerson(result.Person, censusYear); // } // familyResults.Add(simpleFamily); // } // } //} //return new JsonResult //{ // Data = familyResults, // JsonRequestBehavior = JsonRequestBehavior.AllowGet //}; //#endregion ////////////////////////////////////////////////////// filteredResults = results.PersonContainer.GetFamilies().Where(x => x.Id.Contains(databaseId.ToString())).Take(5).ToList(); foreach (Family family in filteredResults) { SimpleFamily simpleFamily = new SimpleFamily(); simpleFamily.Id = family.Id; simpleFamily.CensusYear = searchCensusYear; //simpleFamily.Version = family.Version; simpleFamily.Mother = SimplePerson.CreatePerson(results.PersonContainer.GetPerson(family.MotherId), simpleFamily.CensusYear); simpleFamily.Father = SimplePerson.CreatePerson(results.PersonContainer.GetPerson(family.FatherId), simpleFamily.CensusYear); foreach (RelationshipPointer child in family.Children.ChildPointers) { simpleFamily.Children.Add(SimplePerson.CreatePerson(results.PersonContainer.GetPerson(child.Id), simpleFamily.CensusYear)); } simpleFamily.FindPerson(results); familyResults.Add(simpleFamily); } List<QueryResult> singlePeople = results.Where(x => results.PersonContainer.GetFamilies(x.Person.Id).Count == 0 && x.Person.Id.DatabaseId == databaseId).ToList(); foreach (QueryResult single in singlePeople) { SimpleFamily simpleFamily = new SimpleFamily(); simpleFamily.Id = ""; simpleFamily.CensusYear = searchCensusYear; simpleFamily.IsSingleHouseHold = true; simpleFamily.SinglePerson = SimplePerson.CreatePerson(single.Person, censusYear); simpleFamily.SinglePerson.Selected = true; simpleFamily.FindPerson(results); familyResults.Add(simpleFamily); } return new JsonResult { Data = familyResults, JsonRequestBehavior = JsonRequestBehavior.AllowGet }; }
public Task <IResult <List <Reputation> > > Execute() { return(QueryProcessor.Process <Reputation, IReputationListQuery, ReputationListQuery>(this, context => context.SubuserReputations(this))); }
public void QueryProcessor_TestExecute_FilteredAndOrdered() { //Arrange: var connectionInfo = CreateConnectionInfo(); var registrants = CreateRegistrants(); var webinar = CreateUpcomingWebinars(); var misMatchedRegistrant = new Registrant { Email = "*****@*****.**", WebinarKey = "246810", FirstName = "john" }; registrants.Add(misMatchedRegistrant); var query = CreateFilterQuery(); //set up the fake data: var clientMoq = new Mock<IGoToWebinarClient>(); clientMoq.Setup(client => client.GetUpcomingWebinars(connectionInfo["AccessToken"], connectionInfo["OrganizerKey"])).Returns(webinar); clientMoq.Setup(client => client.GetRegistrants(connectionInfo["AccessToken"], connectionInfo["OrganizerKey"], "1235813")).Returns(registrants); var queryProcessor = new QueryProcessor(connectionInfo, clientMoq.Object); //Act: IEnumerable<DataEntity> entities = queryProcessor.ExecuteQuery(query); //Assert //check the count: Assert.AreEqual(2, entities.Count()); //check the ordering. We specified Descending, so Jim should be at the top, Bob at the bottom. DataEntity jim = entities.First(); Assert.IsTrue(jim.Properties.Any(field => field.Value == "jim")); }
public void QueryProcessor_TestExecuteQuery_UnknownEntityName() { //Test an unknown entity: //Arrange: var connectionInfo = CreateConnectionInfo(); var query = new Query(); query.RootEntity.ObjectDefinitionFullName = "UnkownEntity"; var clientMoq = new Mock<IGoToWebinarClient>(); var queryProcessor = new QueryProcessor(connectionInfo, clientMoq.Object); //Act: IEnumerable<DataEntity> dataEntities = queryProcessor.ExecuteQuery(query); //Assert: Assert.IsNotNull(dataEntities); }
protected EviStorage(IR2RML mapping, ISqlDatabase <TQuery> database) { _queryProcessor = new QueryProcessor <TQuery>(mapping, database); }
public Task <IResult <List <SenderIdentity> > > Execute() { return(QueryProcessor.Process <SenderIdentity, ISenderIdentityListQuery, SenderIdentityListQuery>(this, context => context.SenderIdentities())); }
public VacanciesController(QueryProcessor queryProcessor) { _queryProcessor = queryProcessor; }
public Task <IResult <IpWarmup> > Execute() { return(QueryProcessor.Process <IpWarmup, IIpWarmupSingleQuery, IpWarmupSingleQuery>(this, context => context.IpWarmupByIpAddress(IpAddress))); }
public static QueryProcessor GetQueryHandlers() { QueryProcessor handler = new QueryProcessor(); handler.Subscribe(new GetOrderByIdQueryHandler(ContextFactory.GetContext().Set<Client>())); return handler; }
public Task <IResult <BrandedLink> > Execute() { return(QueryProcessor.Process <BrandedLink, IDefaultBrandedLinkSingleQuery, DefaultBrandedLinkSingleQuery>( this, context => context.DefaultBrandedLink(DomainUrl))); }
public static IQueryProcessor GetQueryProcessor() { var processor = new QueryProcessor(); processor.Subscribe(new QueryHandlerProxy<FetchAllClients, ClientDto[]>()); return processor; }
public async Task OnGetAsync() { 本一覧 = await QueryProcessor.ProcessAsync(new 本DTOQuery(), CancellationToken.None); }