public static string ToCsvString(this object value, ExcelCustomPropertyInfo p) { if (value == null) { return(""); } Type type = null; if (p == null) { type = value.GetType(); type = Nullable.GetUnderlyingType(type) ?? type; } else { type = p.ExcludeNullableType; //sometime it doesn't need to re-get type like prop } if (type == typeof(DateTime)) { if (p == null || p.ExcelFormat == null) { return(((DateTime)value).ToString("yyyy-MM-dd HH:mm:ss")); } else { return(((DateTime)value).ToString(p.ExcelFormat)); } } return(value.ToString()); }
private static void WriteCell(StreamWriter writer, int yIndex, int cellIndex, object value, ExcelCustomPropertyInfo p) { var v = string.Empty; var t = "str"; var s = "2"; if (value == null) { v = ""; } else if (value is string) { v = ExcelOpenXmlUtils.EncodeXML(value.ToString()); } else { Type type = null; if (p == null) { type = value.GetType(); type = Nullable.GetUnderlyingType(type) ?? type; } else { type = p.ExcludeNullableType; //sometime it doesn't need to re-get type like prop } if (Helpers.IsNumericType(type)) { t = "n"; v = value.ToString(); } else if (type == typeof(bool)) { t = "b"; v = (bool)value ? "1" : "0"; } else if (type == typeof(DateTime)) { if(p==null || p.ExcelFormat == null) { t = null; s = "3"; v = ((DateTime)value).ToOADate().ToString(); } else { t = "str"; v = ((DateTime)value).ToString(p.ExcelFormat); } } else { v = ExcelOpenXmlUtils.EncodeXML(value.ToString()); } } var columname = ExcelOpenXmlUtils.ConvertXyToCell(cellIndex, yIndex); //t check avoid format error 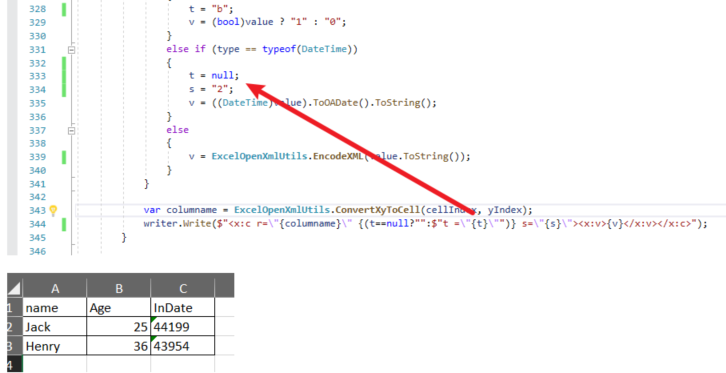 writer.Write($"<x:c r=\"{columname}\" {(t == null ? "" : $"t =\"{t}\"")} s=\"{s}\"><x:v>{v}</x:v></x:c>");